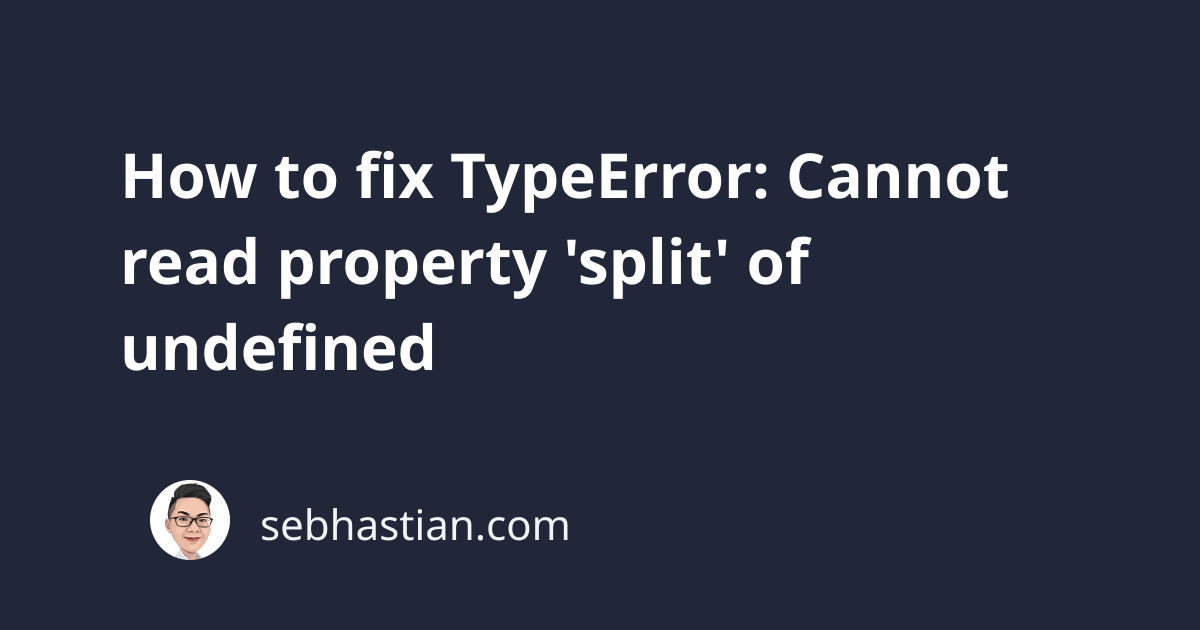
One error you might encounter when running JavaScript code is:
TypeError: Cannot read property 'split' of undefined
This error occurs when you call the split()
method from a variable with undefined
as its value.
Let’s see an example code that causes this error. The following code tries to split the value of the username
variable into an array, using an underscore _
as the separator:
let username = undefined;
let usernameArr = username.split("_");
The split()
method is a method of the String
prototype object, so you can only call the method from a string type variable.
The code below shows the right way to use the split()
method on a string type value:
let username = "Nathan_Sebhastian";
let usernameArr = username.split("_");
console.log(usernameArr);
Output:
[ 'Nathan', 'Sebhastian' ]
Here, the username
variable is a string type value, so the split()
method works without any error.
To prevent this error, you can use an if
statement and check the type of your variable using the typeof
operator:
let username = "Nathan_Sebhastian";
if (typeof username === "string") {
let usernameArr = username.split("_");
console.log(usernameArr);
} else {
console.log("The 'username' variable is not a string");
}
Here, the if
block runs only when the type of the username
variable is a ‘string’. For any other type, JavaScript will run the else
block.
Please note that others might suggest you use a strict not equal !==
operator and check if the type is not equal to undefined
.
I don’t recommend this solution because you can get another kind of error when you have a value that’s not ‘undefined’ or ‘string’.
For example, suppose you have an integer type variable as follows:
let age = 24;
if (typeof age !== "undefined") {
let result = age.split("_");
console.log(result);
}
Here, the age
variable is not undefined
, so the if
block gets executed. But because the variable isn’t a ‘string’, you get an error saying:
TypeError: age.split is not a function
This error occurs because the Number
object doesn’t have the split()
method. So even when the variable is not undefined
, you still encounter an error because the variable isn’t a string.
The same goes when you use the optional chaining operator:
let age = 24;
let result = age?.split("_");
console.log(result);
The optional chaining operator only checks if the value isn’t ‘undefined’ or ’null’. When you pass any other value, the operator will call the chained method.
This is why the best solution to this error is to use the typeof
operator and the strict equality comparison operator ===
to check if the variable’s value is a string type.
Now you’ve learned why the TypeError: Cannot read property ‘split’ of undefined occurs and how to fix it.
I hope this tutorial helps. Happy coding and see you in other tutorials! 👋