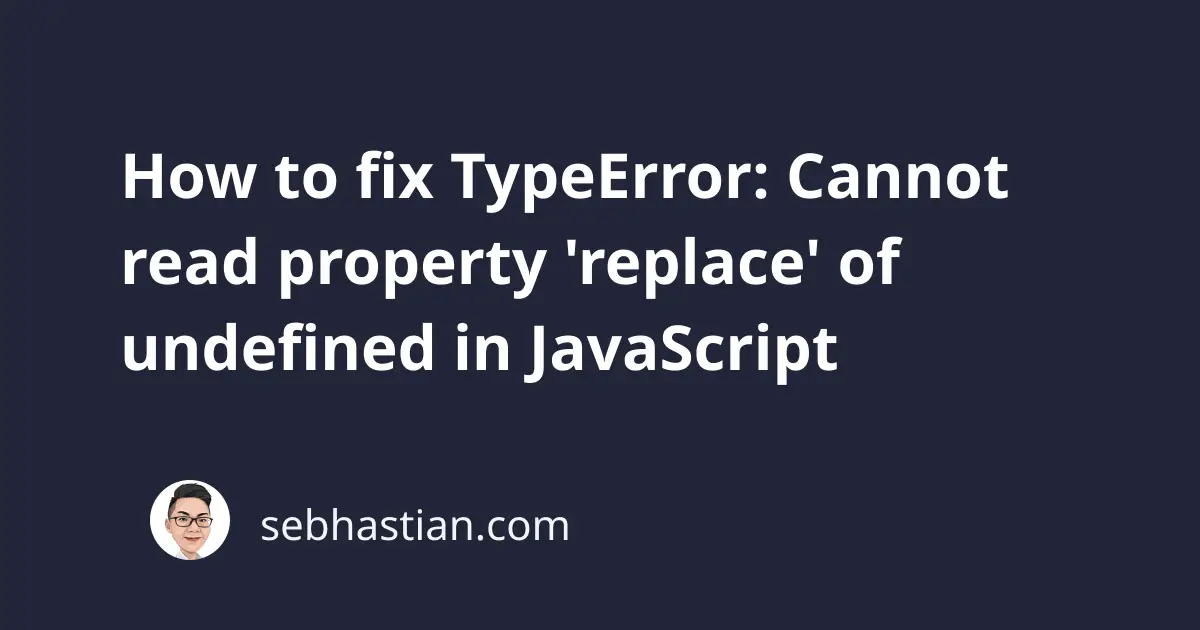
When running JavaScript code, you might see the following error:
TypeError: Cannot read property 'replace' of undefined
or
TypeError: Cannot read properties of undefined (reading 'replace')
Both error messages mean the same thing. You are calling the replace()
method on a variable that has a value of undefined
.
This tutorial will show you an example code that causes this error and how I fix it.
How to reproduce the error
This error occurs anytime you call the replace()
method from an undefined
variable.
Suppose you run the code below:
const myStr = undefined;
const newStr = myStr.replace("old", "new");
console.log(newStr);
Here, you try to replace the string ‘old’ with ’new’ from the variable myStr
.
But since myStr
has the value of undefined
, the error occurs:
TypeError: Cannot read properties of undefined (reading 'replace')
To resolve this error, you need to make sure that you’re not calling the replace()
method from an undefined
value.
There are 4 simple ways you can fix this error. Let’s go over one by one in this tutorial
1. Use an if statement
One way to resolve this error is to check the value of your variable using an if
statement.
You need to check that the value of myStr
is truthy as follows:
const myStr = undefined;
if (myStr) {
const newStr = myStr.replace("old", "new");
console.log(newStr);
}
Here, the if
block runs only when the value of myStr
is truthy (doesn’t return false
).
2. Use the ternary operator
You can also fix the error by checking the type of the variable.
In JavaScript, undefined
is its own type, so you can use the typeof
operator and check if the variable is a ‘string’ as follows:
const myStr = undefined;
const newStr =
typeof myStr === "string" ? myStr.replace("old", "new") : "";
console.log(newStr);
Because the type of the variable isn’t a ‘string’, the ternary operator returns an empty string.
You can also change the value into any other default value you want to use.
3. Use the optional chaining and nullish coalescing operators
As an alternative to the ternary operator, you can use the optional chaining and nullish coalescing operators.
The optional chaining operator only calls the replace()
method when the variable is truthy, else it returns undefined
.
The nullish coalescing operator is added to serve as a fallback value, like the colon :
operator in the ternary operator:
const myStr = undefined;
const newStr = myStr?.replace("old", "new") ?? "Undefined string";
console.log(newStr);
Here, the fallback value is ‘Undefined string’ instead of an empty string.
4. Use the logical OR || operator to add a fallback value
You can also use the logical OR ||
operator to add a fallback value, replacing the nullish coalescing operator:
const myStr = undefined;
const newStr = myStr?.replace("old", "new") || "Undefined string";
console.log(newStr);
Here, the logical OR works like the nullish coalescing operator. When both expressions are truthy, it returns the value on the left side; you can set the fallback value on the right side.
Conclusion
The TypeError: Cannot read property 'replace' of undefined
occurs when the replace()
method is called from a variable that has a value of undefined
.
To fix this error, you need to check on the variable from which you called the replace()
method. Make sure that the variable is not undefined
or null
.
I hope this tutorial helps. Happy coding! 🙌