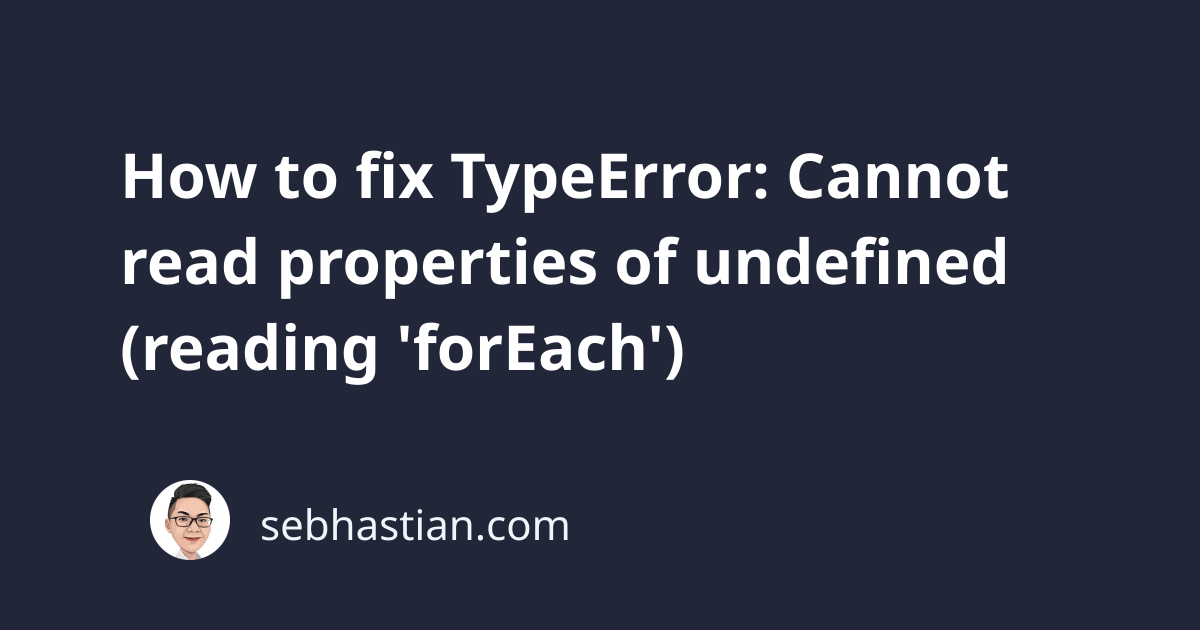
The error “Cannot read property forEach of undefined” occurs when you try to call the forEach()
method on an undefined
value.
In the latest JavaScript version, the error is reworded as “Cannot read properties of undefined (reading ‘forEach’)”. I feel the old error message is more clear.
One example code that causes the error is as follows:
undefined.forEach(element => {
console.log(element);
});
Here, you can see clearly that the forEach
method is called on an undefined
value, causing the error.
Most of the time though, you might trigger this error unintentionally, such as when calling forEach
after declaring a variable like this:
let arr = [1, 2]
[3, 4].forEach(element => {
console.log(element);
});
When running the code above, you’ll get this error:
[3, 4].forEach(element => {
^
TypeError: Cannot read properties of undefined (reading 'forEach')
This is because the declaration of the arr
variable above the array [3, 4]
causes JavaScript to see the declaration as let arr = [1, 2][3, 4].forEach..
The two code lines are executed as one, and this causes the error. To resolve it, you need to add a semicolon after declaring the variable as follows:
let arr = [1, 2];
[3, 4].forEach(element => {
console.log(element)
});
The semicolon separates the first line and the rest, and now you can run the forEach()
method without causing the error.
Use Array.isArray() to check the variable value
To make sure that you’re not calling the forEach
method on an undefined
value, you need to check on the value of the variable using an if
statement as follows:
let arr = [1, 2];
if (Array.isArray(arr)) {
arr.forEach(element => {
console.log(element);
});
}
The Array.isArray()
method is used to check whether the value you passed is an array. This check will make sure that you only call the forEach
method on an array.
Using the arrow function and this inside an object
Another example that can cause this error is when you have a function inside an object as follows:
const myObj = {
numbers: [1, 2, 3, 4],
printNumbers: () => {
this.numbers.forEach(number => {
console.log(number);
});
},
};
myObj.printNumbers();
If you run the code above, you get this error:
this.numbers.forEach((number) => {
^
TypeError: Cannot read properties of undefined (reading 'forEach')
This is because the this
keyword in the context above points to the global scope. The arrow function syntax doesn’t create a locally scoped context, so this
points to the window
object instead of the current object.
To resolve this error, you need to use the old function()
syntax as follows:
const myObj = {
numbers: [1, 2, 3, 4],
printNumbers: function() {
this.numbers.forEach(number => {
console.log(number);
});
},
};
myObj.printNumbers();
Now if you run the code again, the printNumbers()
method should work and the error is resolved.
Conclusion
The error “Cannot read properties of undefined (reading ‘forEach’)” happens when you call the forEach
method from an undefined
value.
The forEach
method is only available on array and array-like objects (map, set, nodeList, etc.) so you must not call it from an undefined
value.
I hope the solutions provided in this tutorial help you solve the error. Happy coding! 👍