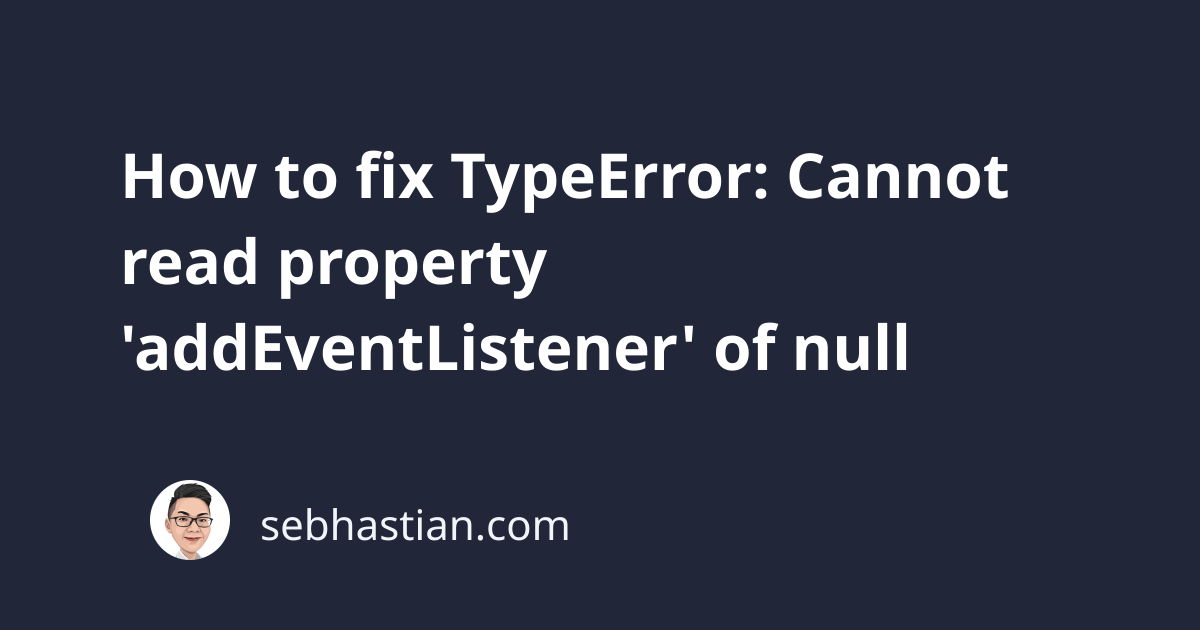
One error you might encounter when running JavaScript code is:
Uncaught TypeError: Cannot read properties of null (reading 'addEventListener')
This error occurs when you try to call the addEventListener()
method from a null
value.
Most likely, you tried to get a DOM element using JavaScript selector methods like document.getElementById()
and document.querySelector()
but the element doesn’t exist.
The selector methods will return null
when the element you select isn’t found. In the following example, we tried to get an element that has the id
attribute of tag
:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Nathan Sebhastian</title>
</head>
<body>
<button id="btn">Sign up</button>
<script>
let btnEl = document.getElementById("tag");
btnEl.addEventListener("click", () => alert("You clicked a button!"));
</script>
</body>
</html>
Note that the <button>
element in the example has an id
attribute with btn
as its value. Because there’s no element that has the tag
id, the document.getElementById()
method returns null
.
Next, the addEventListener()
method is called on the btnEl
variable, which has a null
value.
This causes the following error:
Uncaught TypeError: Cannot read properties of null (reading 'addEventListener')
at index.html:11:13
To resolve this error, you need to make sure that you’re calling the addEventListener()
method on a valid Element
object.
The key is to check on the variable that receives the value returned by the selector method you used. There are 2 easy ways to do it:
- Use an
if
statement - Use an optional chaining operator
Let’s see a quick example of how these solutions can be applied.
1. Using an if statement
You can use an if
statement to avoid calling the addEventListener()
method on a null
object. See the following example:
let btnEl = document.getElementById("tag");
if (btnEl) {
btnEl.addEventListener("click", () => alert("You clicked a button!"));
} else {
alert("The element is not found!");
}
Here, JavaScript calls the addEventListener()
method only when the btnEl
variable contains a truthy value (a value that evaluates to true
)
Selector methods return either a null
or a valid Element
object, so the if
block will run only when the variable is not null
.
When the element is null
, the else
block will run, alerting you that the element isn’t found.
2. Using an optional chaining operator
You can also use the optional chaining operator ?.
to prevent JavaScript from calling the addEventListener()
method from a null
value.
Here’s an example of using the optional chaining operator:
let btnEl = document.getElementById("tag");
btnEl?.addEventListener("click", () => alert("You clicked a button!"));
The optional chaining operator evaluates the object from which you called a method or accessed a property. When the object is null
or undefined
, then the operator skips the operation and returns undefined
instead of throwing an error.
If you need to keep the returned value, you can assign the code line to a variable as follows:
let result = btnEl?.addEventListener("click", () => alert("You clicked a button!"));
console.log(result);
Please note that the addEventListener()
method returns nothing, so the result
variable will always be undefined
no matter if the method gets called successfully.
Conclusion
The error Cannot read property 'addEventListener' of null
occurs when you try to call the addEventListener()
method from a null
value.
The most common cause of this error is calling the method from a variable that holds the return value of a selector method like document.getElementById()
, which returns null
when the element to get isn’t found.
To prevent this error, you can use an if
statement or an optional chaining operator. Which one to use? If you need to perform additional operations when the element isn’t found, use an if
statement.
Otherwise, use an optional chaining operator because it’s shorter and more concise. Note that you can’t know whether the method gets called or not because the return value of addEventListener()
is always undefined
.
I hope this tutorial helps you write better code. See you in the next tutorial! 👋