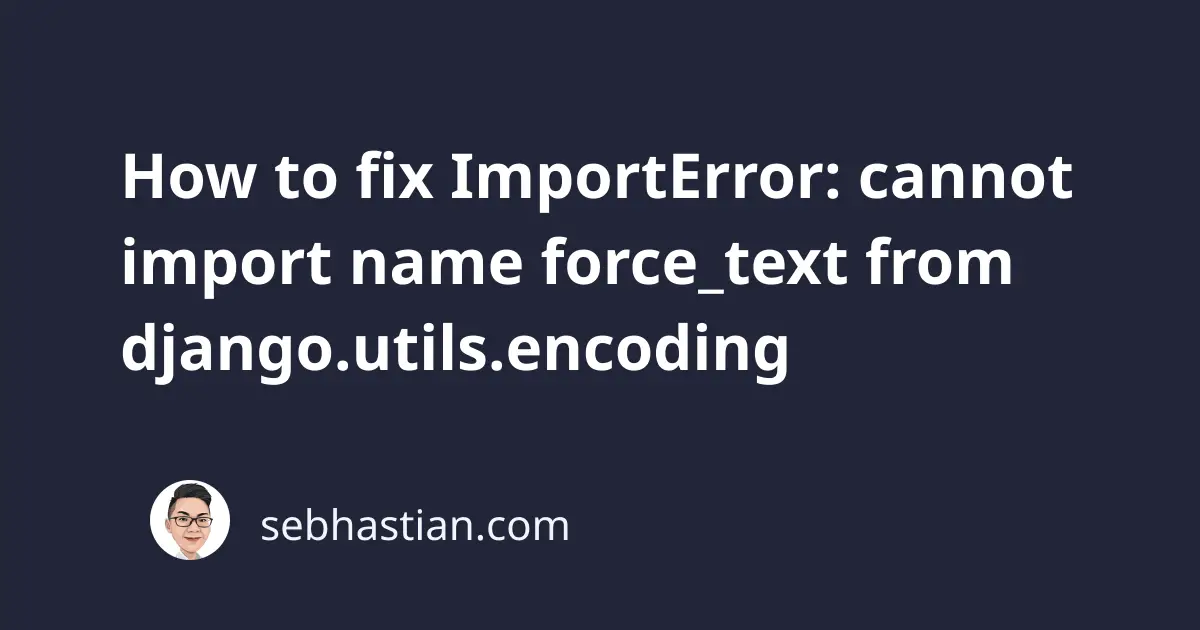
If you use Django to develop web applications with Python, you might see the following error:
Result: Failure Exception:
ImportError: cannot import name 'force_text' from 'django.utils.encoding'
After doing some research, I found that this error occurs because the force_text
function has been deprecated1 and removed in Django version 4.
The force_text
function is an alias for the force_str
function, and it was kept in Django 3 to support Python 2.
When Django 4 was released, the force_text
function is removed, so you need to use force_str
directly.
Here’s the code that causes this error:
from django.utils.encoding import force_text
To fix this error, you need to replace force_text
with force_str
as follows:
from django.utils.encoding import force_str
# ...
str_res = force_str(s)
Many Django-related packages also use force_text
in their code. You need to upgrade them to the latest versions to solve this error.
Some of them are:
- Graphene-Django
- Django Elasticsearch DSL
- Django Smart Selects
- djangorestframework-simplejwt
If you used any of these packages in your Django project, then you need to upgrade them to the latest versions.
Use the following pip
command to upgrade these packages:
pip install --upgrade graphene-django
pip install --upgrade django-elasticsearch-dsl
pip install --upgrade djangorestframework-simplejwt
pip install --upgrade django-smart-selects
Once you have the latest version of the third-party libraries, you shouldn’t see this error anymore.
You can also use this command to upgrade all dependencies installed on your site-packages/
folder to the latest versions:
pip freeze | grep -v '^\-e' | cut -d = -f 1 | xargs -n1 pip install --upgrade
#For pip3:
pip3 freeze | grep -v '^\-e' | cut -d = -f 1 | xargs -n1 pip3 install --upgrade
Also if you have a requirements.txt
file, then you need to replace all ==
, <=
, and <
symbols with >=
.
If you have something as follows:
graphene>=2.1,<3
graphene-django>=2.1,<3
graphql-core>=2.1,<3
graphql-relay==2.0.1
django-filter>=2
django<=3.2.0
Then upgrade it to:
graphene>=2.1
graphene-django>=2.1
graphql-core>=2.1
graphql-relay>=2.0.1
django-filter>=2
django>=3.2.0
The >=
symbol tells pip
to install the specified version or above. Save the modified requirements.txt
file and run the command:
pip install -r requirements.txt --upgrade
Once the installation is finished, try running your code again.
Alternatively, you can also downgrade the installed Django version, but keep in mind that this is a temporary solution until you’re ready to migrate your project to the latest Django version.
To downgrade Django version, you can run the following command:
pip install 'django<4' --force-reinstall
The above command tells pip
to install the latest Django below version 4, which is Django v3.2.17.
In this Django version, the force_text
function is still available so you won’t receive the error.
Conclusion
The ImportError: cannot import name 'force_text' from 'django.utils.encoding'
occurs because Django removed the force_text
function when version 4 was released.
Based on my experience and research, you need to replace the import statement for force_text
with force_str
to resolve this error.
I hope this tutorial is helpful. Happy coding! 👍