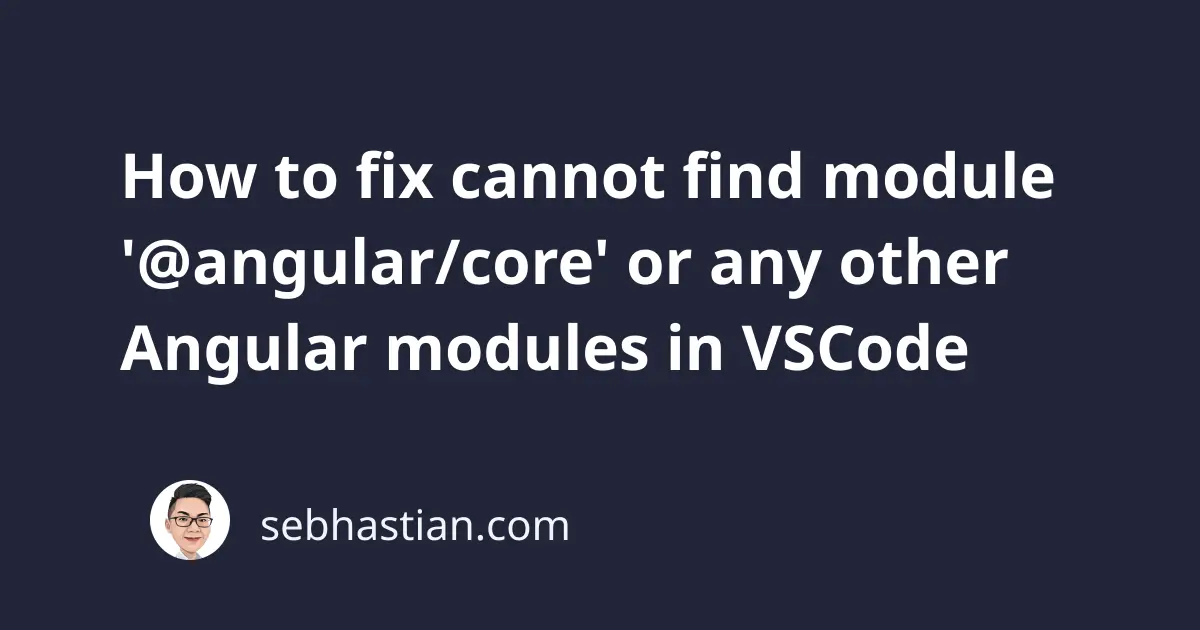
Sometimes, you get an error marked by red squiggly lines in VSCode, saying that it Cannot find module ‘@angular/core’ as shown below:
When you see this error, the first thing you need to do is try to run the Angular application using the ng serve
or npm start
command.
If you can compile and run the application just fine, then the error occurs because VSCode has a cache that makes it think the Angular modules haven’t been installed yet.
If you can’t run the application, then you need to clean your npm_modules/
folder and run a fresh npm install
command:
# Delete node_modules folder for Mac/Linux
rm -rf node_modules
rm -f package-lock.json
rm -f yarn.lock
# Delete node_modules folder for Windows
rd /s /q "node_modules"
del package-lock.json
del -f yarn.lock
# with NPM
npm install
# or with YARN
yarn install
Once the installation process is finished, run the application again. The error should disappear now.
Set the baseUrl
config to src
in tsconfig.json
file
If you still see this error, then you can try to set the baseUrl
config to src
in your tsconfig.json
file.
Sometimes, the reason is that VSCode IDE is unable to resolve the base URL of your application. This makes the IDE unable to resolve the path to imported components and causes the error.
You can help the IDE pick the right base URL as follows:
{
"compilerOptions": {
"baseUrl": "src"
//...
}
}
Save the file, then restart VScode so the changes take effect.
Uninstall Deno for Visual Studio Code
If the above solution doesn’t work, then check if you have Deno for Visual Studio Code extension installed on your IDE.
The Deno support extension is known to cause this error, so you need to uninstall or disable it when running an Angular application.
After you uninstall or disable the extension, restart VSCode so the changes take effect.
Clean your npm cache
If the error isn’t resolved, then try clearing your npm cache and reinstall the dependencies as follows:
# clean npm cache
npm cache clean --force
# install packages
npm install
Clearing the cache should help npm grab a fresh package from its repository.
Check your package.json file and make sure there’s angular/core listed
If you still see the error, then open your package.json
file and check if the angular
modules are listed as dependencies.
A correct package.json
file should have the dependencies
and devDependencies
specified as follows:
{
// other details
"dependencies": {
"@angular/animations": "^15.1.0",
"@angular/common": "^15.1.0",
"@angular/compiler": "^15.1.0",
"@angular/core": "^15.1.0",
"@angular/forms": "^15.1.0",
"@angular/platform-browser": "^15.1.0",
"@angular/platform-browser-dynamic": "^15.1.0",
"@angular/router": "^15.1.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.12.0"
},
"devDependencies": {
"@angular-devkit/build-angular": "^15.1.5",
"@angular/cli": "~15.1.5",
"@angular/compiler-cli": "^15.1.0",
"@types/jasmine": "~4.3.0",
"jasmine-core": "~4.5.0",
"karma": "~6.4.0",
"karma-chrome-launcher": "~3.1.0",
"karma-coverage": "~2.2.0",
"karma-jasmine": "~5.1.0",
"karma-jasmine-html-reporter": "~2.0.0",
"typescript": "~4.9.4"
}
}
As you can see, there are lots of dependencies to run an Angular application.
Instead of installing them one by one, you can try to create a new Angular application using the ng new
command:
ng new <project name>
Once created, run the application with the ng serve
command. If you can run the new application without error, then try copying your old application code into the new application.
Adjust the dependencies as needed and see if you can run the old application here.
Conclusion
The error cannot find module '@angular/core'
occurs when the @angular/core
package can’t be found on your computer. To resolve the error, try installing the package with the npm install
command.
If the package is installed but the error persists, then set the baseUrl
parameter in tsconfig.json
file and restart VSCode to see if the error disappears.
I hope this tutorial helps. Happy coding!