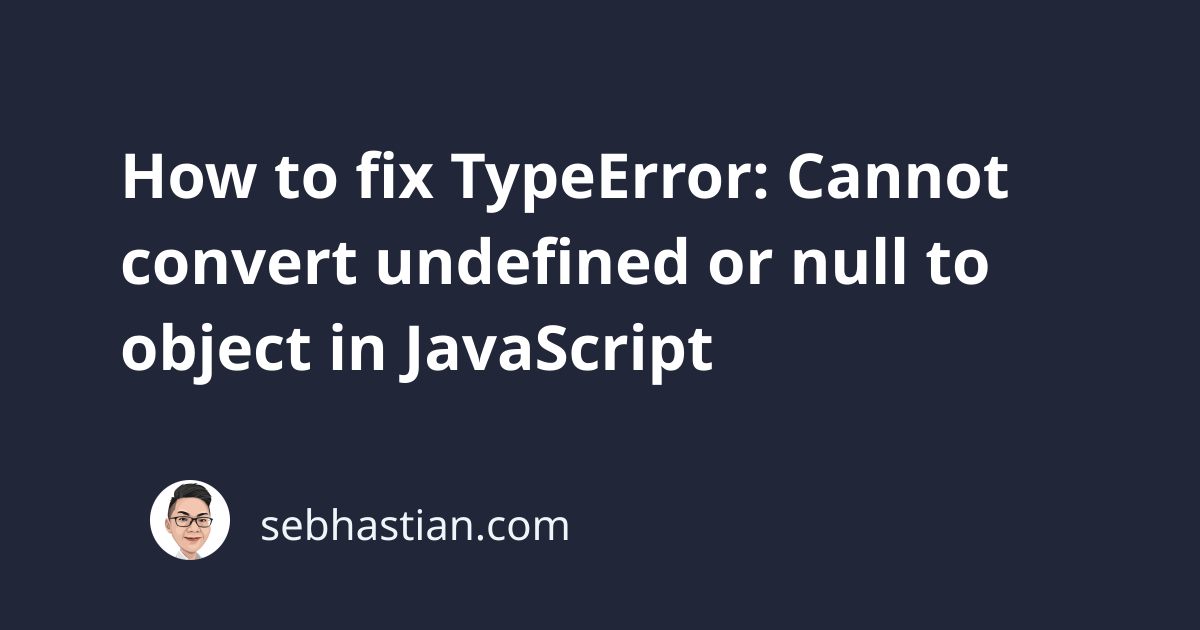
One error you might encounter when running JavaScript code is:
TypeError: Cannot convert undefined or null to object
This error occurs when you pass either null
or undefined
to a function that expects an object.
Usually, it happens when you call the Object
methods like Object.keys()
, Object.values()
, and Object.assign()
.
Let’s see an example that causes this error and how you can fix it.
How to reproduce the error
To cause this error, you need to pass null
or undefined
to a function that requires an object.
The following example all cause the same error:
Object.keys(null);
Object.values(undefined);
Object.assign(null, {});
The above methods expected an object as its parameter, but both null
and undefined
can’t be converted into an object. Hence the error.
How to fix this error
To resolve this error, you need to make sure that you’re not passing null
or undefined
to the methods.
Most likely you’re passing a variable to the above methods. One way to check if the variable is null
or undefined
is to use an if
statement as follows:
let myObj = {
name: "John",
};
if (myObj) {
// call the method here
Object.assign(myObj, { age: 25 }); // ✅
}
console.log(myObj); // { name: 'John', age: 25 }
The error doesn’t appear when you run the code above because the if
statement checks that the myObj
variable is not null
or undefined
before calling the Object.assign()
method.
You can also use a ternary operator and choose to call the method to replace the if
statement as follows:
let myObj = undefined;
let newObj = myObj ? Object.assign(myObj, { age: 25 }) : {};
console.log(newObj); // {}
Because myObj
is undefined, the Object.assign()
method didn’t get called and the empty object is assigned to the newObj
variable.
Another way to fix this error is to provide a fallback value when you define the argument to the method.
You can use the logical OR ||
operator and add a fallback value as follows:
let myObj = {
name: "John",
};
const objKeys = Object.keys(myObj || {});
Here, an empty object {}
serves as the fallback value when the value of myObj
variable is null
or undefined
.
And that’s how you fix the TypeError: Cannot convert undefined or null to object
in JavaScript.
I hope this tutorial helps. See you in other tutorials! 👋