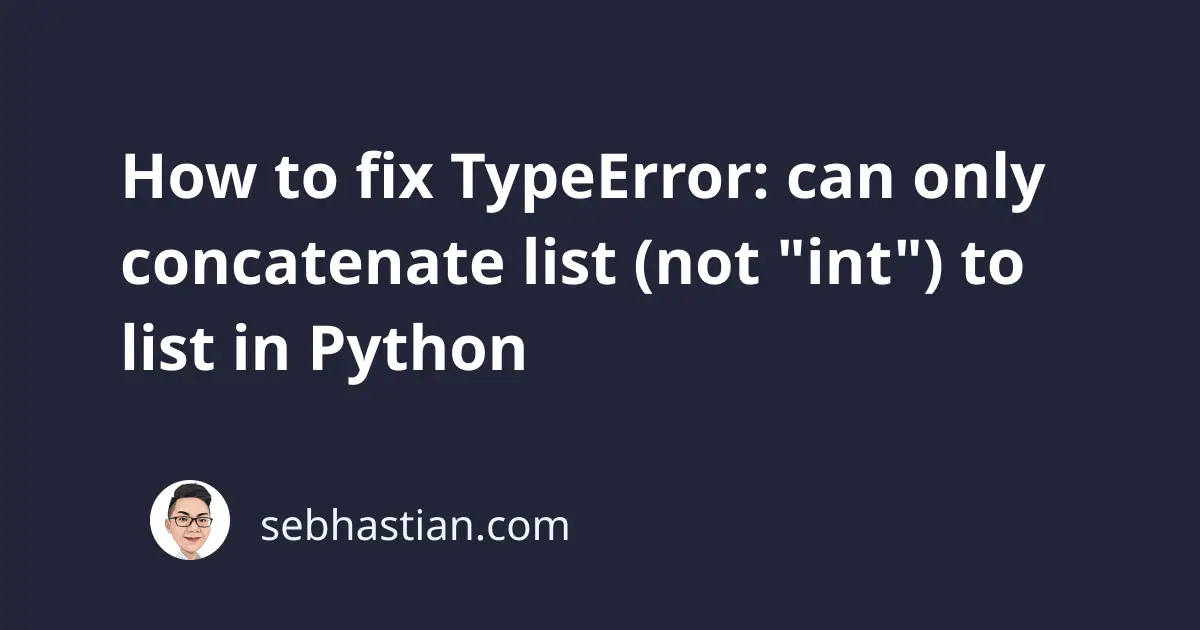
One error that you might get when working with Python code is:
TypeError: can only concatenate list (not "int") to list
This error occurs when you attempt to concatenate an integer value to a list. Most probably, you’re trying to add an integer to a list with the +
operator.
This tutorial will show you an example that causes this error and how to fix it.
How to reproduce this error
Suppose you have a list that contains integer numbers, then you add a single integer number to that list as follows:
my_integers = [1, 2, 3]
new_list = my_integers + 4
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
new_list = my_integers + 4
~~~~~~~~~~~~^~~
TypeError: can only concatenate list (not "int") to list
This error occurs because Python only allows you to add a list to another list. You can’t concatenate an integer into a list as in the example.
How to fix this error
To resolve this error and add the integer to the list, you need to call the append()
method of the list object.
The append()
method adds an item to the list from the end as shown below:
my_integers = [1, 2, 3]
my_integers.append(4)
print(my_integers) # [1, 2, 3, 4]
Please note that the append()
method modifies the original list.
If you want to keep the original list unchanged, you can put the integer number into a list, then add the list to the original list as follows:
my_integers = [1, 2, 3]
new_list = my_integers + [4]
print(my_integers) # [1, 2, 3]
print(new_list) # [1, 2, 3, 4]
As you can see, the my_integers
list is now preserved. The +
operator works fine when you concatenate two lists.
Conclusion
The TypeError: can only concatenate list (not "int") to list
occurs when you try to add an integer into a list.
To resolve this error, you need to use the append()
method to add an integer to a list.
If you want to keep the original list, you need to wrap the integer in a list and add the list to the original list.
I hope this tutorial is helpful. Thanks for reading! 👍