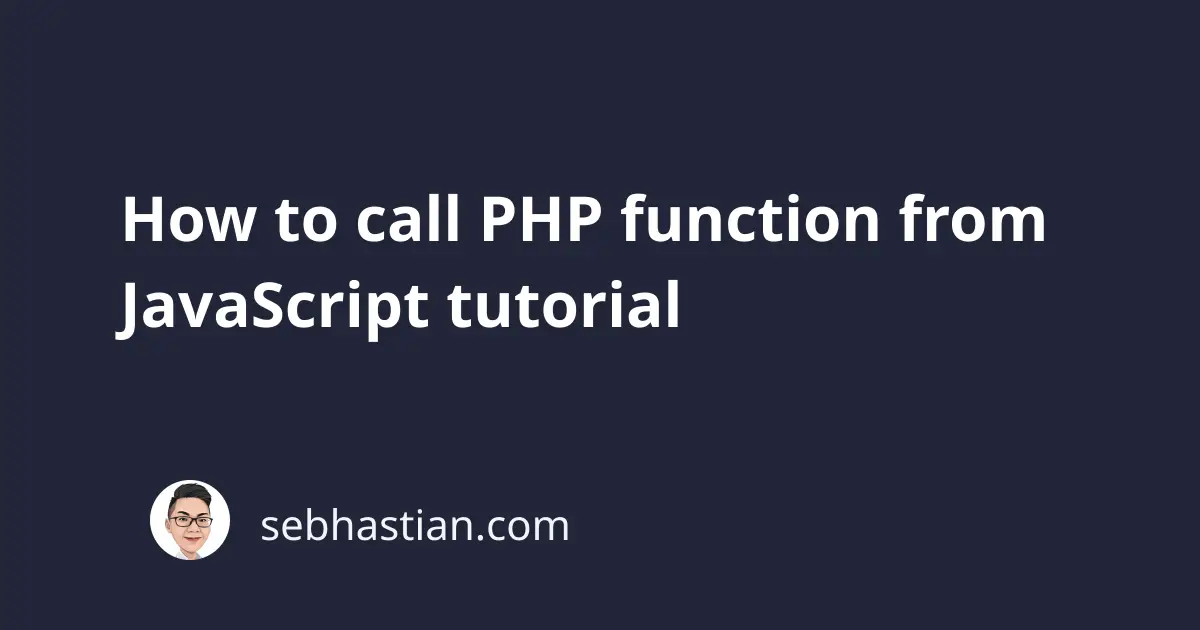
There are two ways of calling a PHP function from JavaScript depending on your web project’s architecture:
- You can call PHP function inline from the
<script>
tag - You can use
fetch()
JavaScript method and send an HTTP request to the PHP server
This tutorial will help you learn both ways easily. Let’s start with calling an inline PHP function
Call PHP function from JavaScript inline
If you’re using PHP files to render your web pages, then you can call the PHP function from JavaScript by calling the PHP echo()
function.
Suppose you have an index.php
file with the following content:
<?php
function add($x, $y){
return $x + $y;
}
?>
Then, you write your HTML content right below the function as follows:
<?php
function add($x, $y){
return $x + $x;
}
?>
// Other HTML tags omitted for clarity
<body>
<h1 id="header"></h1>
</body>
You can include a <script>
tag inside the <body>
tag that calls on PHP function as follows:
<body>
<h1 id="header"></h1>
<script>
const result = <?php echo add(5, 3)?> ;
document.getElementById("header").innerHTML = result;
</script>
</body>
When you open your index.php
file from the browser, you will see the HTML rendered as follows:
<body>
<h1 id="header">8</h1>
<script>
const result = 8;
document.getElementById("header").innerHTML = result;
</script>
</body>
Any PHP code that you include inside your HTML page will be processed on the server-side before being served to the browser.
When you call a PHP function inline from JavaScript as shown above, you can’t use dynamic values from user input.
If you want to call PHP function as a response to user action, then you need to send HTTP request from JavaScript to the PHP file. Let’s learn that next.
Call PHP function from JavaScript over HTTP request
To send HTTP request from JavaScript to PHP server, you need to do the following:
- separate your PHP file from your HTML file
- Call the PHP function over an HTTP request using
fetch()
JavaScript method
First, separate your PHP function in a different file. Let’s call the file add.php
and put the following content inside it:
<?php
$x = $_POST['x'];
$y = $_POST['y'];
echo $x + $y;
?>
Next, create an index.html
file in the same folder where you created the add.php
file and put the following content inside it:
<body>
<button id="add">Add 5 + 3</button>
<div id="result"></div>
<script>
let btn = document.getElementById("add");
let x = 5;
let y = 3;
btn.addEventListener("click", function(){
fetch("http://localhost:8000/add.php", {
method: "POST",
headers: {
"Content-Type": "application/x-www-form-urlencoded; charset=UTF-8",
},
body: `x=${x}&y=${y}`,
})
.then((response) => response.text())
.then((res) => (document.getElementById("result").innerHTML = res));
})
</script>
</body>
The fetch()
method will be executed each time the <button>
element is clicked. JavaScript will send a POST
request to the PHP server and write the response inside the <div id="result">
element.
In the code above, I used the full URL of my add.php
, which is located at http://localhost:8000/add.php
. You need to change the address to your actual PHP file location.
Once your files are ready, open the index.html
file from the browser. Please make sure that you’re opening the HTML file from the same server that serve your PHP files to avoid CORS issues.
You can run a local PHP server using php -s localhost:8000
command and open localhost:8000/index.html
from the browser to see your HTML page.
When you click on the button, the fetch()
method will be executed and JavaScript will put the response inside the <div>
element.
The resulting HTML would be as follows:
<body>
<button id="add">Add 5 + 3</button>
<div id="result">8</div>
<script>
// omitted for clarity...
</script>
</body>
Now that your code is working, you can add <input>
elements to the HTML page and assign the values that the user puts in those elements to x
and y
variables. The PHP function should be able to add the dynamic values correctly
And those are the two ways you can call PHP function from JavaScript. More often, you’d want to separate the PHP files from your JavaScript files and call PHP function using HTTP requests.
The same pattern also works when you’re developing web app using modern PHP framework like Laravel and modern JavaScript libraries like React and Vue.
You can use the fetch()
method and send a POST
request to the right Laravel API route that you created and send the right parameters.