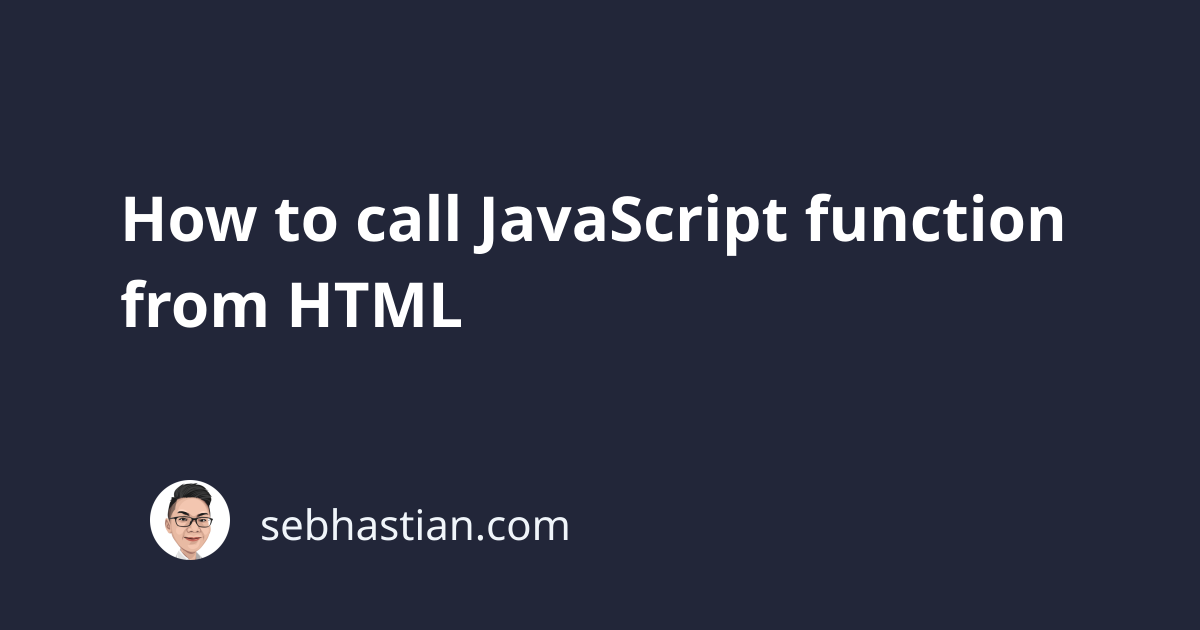
You can call and run a JavaScript function from HTML in several ways.
This tutorial will help you learn the three methods mentioned above.
Calling JavaScript function on HTML page load
You can call and run a JavaScript function immediately after your HTML page has loaded by listening for the load
event.
First, you need to create the function that you want to run from your HTML page. Let’s create a test()
function that will call the alert()
method as follows:
<body>
<h1>Call JavaScript function from HTML</h1>
<script>
function test() {
alert("The function 'test' is executed");
}
</script>
</body>
Then, you need to add JavaScript code that will listen to the load
event from the window
object.
Once the load
event is triggered, call the test()
function as shown below:
window.addEventListener('load', event => {
test();
});
Alternatively, you can use the onload
event handler as follows:
window.onload = event => {
test();
};
Add the code above just below the test()
function definition and you’re good to go:
<body>
<h1>Call JavaScript function from HTML</h1>
<script>
function test() {
alert("The function 'test' is executed");
}
window.addEventListener('load', event => {
test();
});
</script>
</body>
Once your HTML page has finished loading, the load
event will be triggered and the test()
function will be called.
Next, let’s see how you can call a JavaScript function when a button has been clicked.
Call a JavaScript function from an HTML button click event
To call a JavaScript function from a button click, you need to add an event handler and listen for the click
event from your <button>
element.
You can the id
attribute to the <button>
so that you can fetch it using the document.getElementById()
method.
For example, suppose you have the following HTML document:
<body>
<button id="btn">Click me</button>
</body>
You can fetch the element using the document.getElementById()
method, then add the addEventListener()
method to the button as shown below:
let btn = document.getElementById("btn");
btn.addEventListener('click', event => {
test();
});
The full code is as follows:
<body>
<button id="btn">Click me</button>
<script>
function test() {
alert("The function 'test' is executed");
}
let btn = document.getElementById("btn");
btn.addEventListener('click', event => {
test();
});
</script>
</body>
Now once you click on the button, the test()
function will be executed and the alert box will be displayed in the browser.
Alternatively, you can also add the onclick
attribute to the <button>
as shown below:
<body>
<button onclick="test()">Click me</button>
<script>
function test() {
alert("The function 'test' is executed");
}
</script>
</body>
By adding the onclick
attribute, you don’t need to use the document.getElementById()
method and attach an event handler to the button.
Call a JavaScript function from HTML form submit event
To call and run a JavaScript function from an HTML form submit
event, you need to assign the function that you want to run to the onsubmit
event attribute.
Take a look at the following example:
<body>
<form onsubmit="test()">
<label for="username">Enter username:</label>
<input type="text" name="username" id="username" />
<input type="submit" />
</form>
<script>
function test() {
alert(`The function 'test' is executed`);
}
</script>
</body>
By assigning the test()
function to the onsubmit
attribute, the test()
function will be called every time the form is submitted.
Conclusion
The above methods are the three most common methods that you can use to call JavaScript function from HTML.
Because the onclick
attribute is a global HTML attribute, you can actually use it in all HTML tags to call a JavaScript function.
For example, here’s how you can call the test()
method from an <h1>
, a <div>
, or a <p>
tag:
<body>
<h1 onclick="test()">Heading Block</h1>
<div onclick="test()">This is a div block</div>
<p onclick="test()">This is a p block</p>
<script>
function test() {
alert("The function 'test' is executed");
}
</script>
</body>
The onclick
attribute assigned to the tags above is valid and the test()
function will be called each time any one of them is clicked.