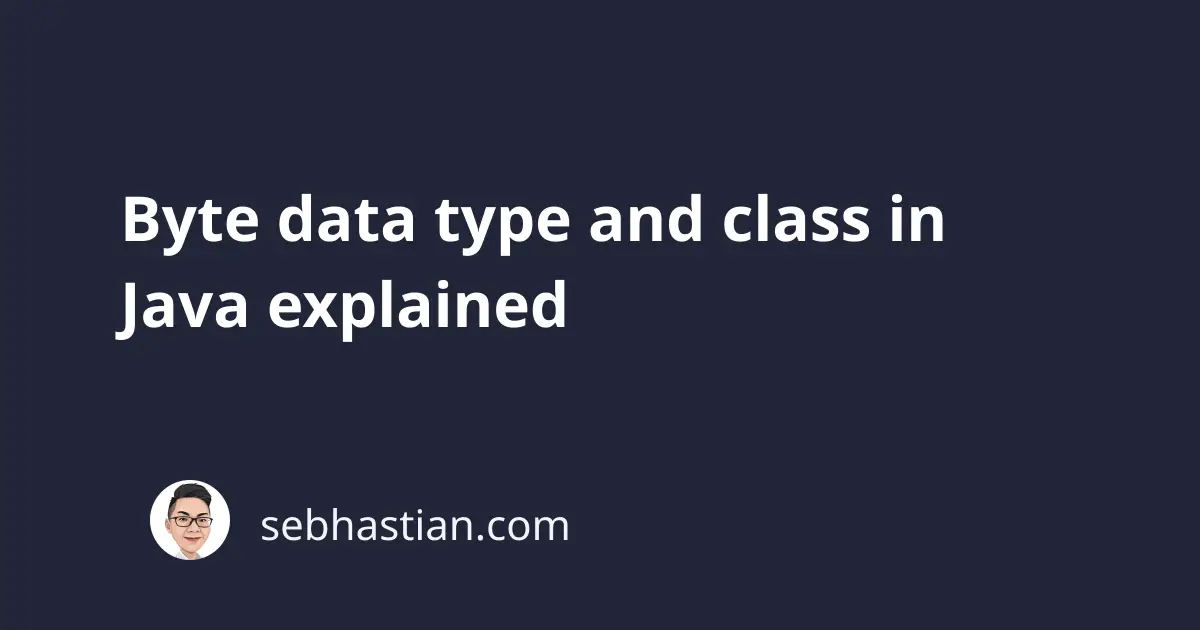
The byte
type is the smallest primitive integer type available in Java. The type is based on an 8-bit signed two’s complement integer, which means it can store an integer value between -128
and 127
.
Here’s how you declare a variable of byte
type in Java:
class Example {
public static void main(String[] args) {
byte n = 8;
System.out.println(n); // 8
}
}
When you put an integer higher than 127
or lower than -128
, Java will throw an incompatible types
error:
class Example {
public static void main(String[] args) {
byte n = 128; // incompatible types: possible lossy conversion from int to byte
System.out.println(n);
}
}
Because byte
is an integer type, you can do the usual arithmetic operations on byte
type variables as follows:
class Example {
public static void main(String[] args) {
byte n = 27;
byte b = 2;
System.out.println(n+b); // 29
}
}
The byte
type also has the Byte
class, which wraps the primitive type in an object
, providing useful methods and constants to manipulate the byte
type data.
Byte class in Java
The Byte
class allows you to create a Byte
object, allowing you to manipulate a byte
data using the following available methods:
byteValue()
- Returns thebyte
value from the currentByte
instancecompareTo(Byte anotherByte)
- Compare twoByte
objects numerically. Returnsint
value of0
when they are equal, less than0
whenanotherByte
is larger, and greater than0
whenanotherByte
is smallerdecode(String nm)
- Decodes aString
into aByte
doubleValue()
- Returns the value of thisByte
as adouble
typeequals(Object obj)
- Compares theByte
instance to anotherObject
. Returnsboolean
value oftrue
orfalse
floatValue()
- Returns the value of thisByte
as afloat
typeintValue()
- Returns the value of thisByte
as anint
typelongValue()
- Returns the value of thisByte
as along
typeparseByte(String s)
- Parses thestring
argument as a signed decimalbyte
toString()
- Returns aString
object representing the specifiedbyte
For example, you can turn a byte
type into a String
object by using the toString()
method as follows:
class Example {
public static void main(String[] args) {
Byte n = new Byte("102");
String str = n.toString();
System.out.println(str); // 102
}
}
And that’s how both byte
type and Byte
class work in Java.