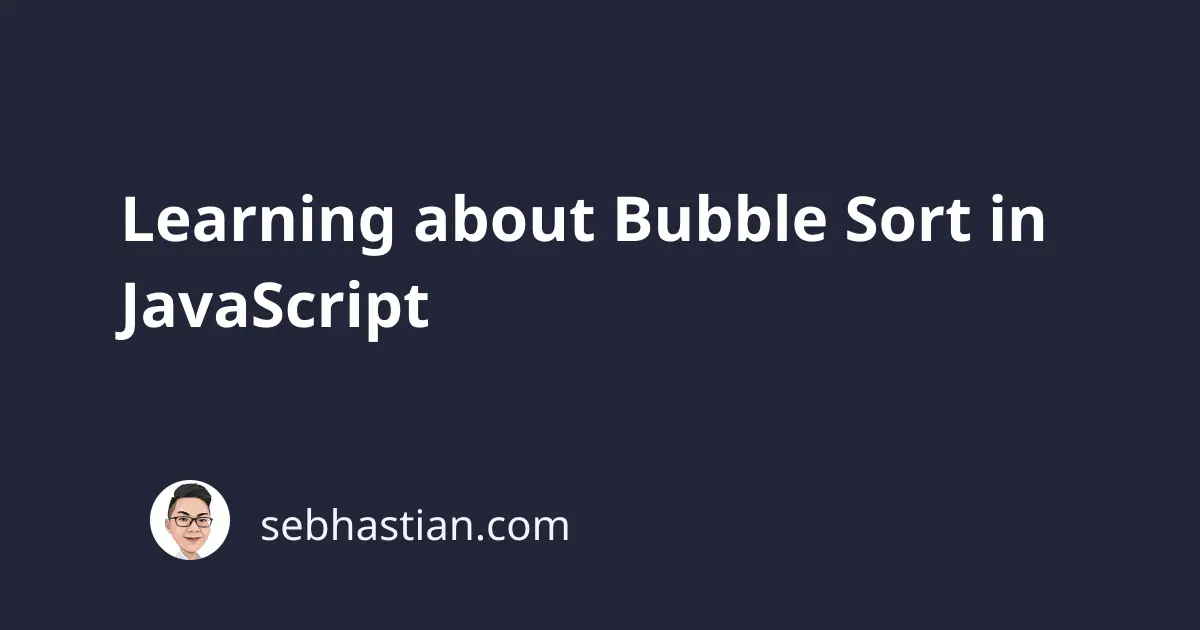
Bubble Sort is a method to sort some list of elements by comparing two of the closest elements from left to right, one at a time. If the element on the left side is greater than the right side, then the elements will be swapped
When implemented correctly, the greater elements usually will “bubble up” to the right place, which is at the top right.
Here’s a diagram to show you how Bubble Sort works:
Implementing Bubble Sort in JavaScript
First, create a function that accepts an array as follows:
function bubbleSort(arr) {
return arr;
}
Next, you need to create a variable the identifies whether the elements are sorted or not. Let’s call this variable sorted
initialized with false
as its value:
function bubbleSort(arr) {
let sorted = false;
return arr;
}
Then, you need to create a while
loop that will execute the sorting code as long as the value of sorted
is false
. You need to change the value of sorted
to true
right below the while
statement:
function bubbleSort(arr) {
let sorted = false;
while (!sorted) {
sorted = true;
// ...
}
return arr;
}
As for the sorting code itself, you need to create a for
loop that will .. array length:
function bubbleSort(arr) {
let sorted = false;
while (!sorted) {
sorted = true;
for (i = 0; i < arr.length; i++) {
}
}
return arr;
}
For the sorting code itself, you need to create an if
statement that compares whether the current index element (arr[i]
) is greater than the element next to it (arr[i+1]
). When it is, swap the elements and change sorted
to false
to signify that a swap has happened:
function bubbleSort(arr) {
let sorted = false;
while (!sorted) {
sorted = true;
for (i = 0; i < arr.length; i++) {
if (arr[i] > arr[i + 1]) {
// swap the elements
let tmp = arr[i];
arr[i] = arr[i + 1];
arr[i + 1] = tmp;
// end swapping
sorted = false;
}
}
}
return arr;
}
Because the elements triggered the swapping and the sorted
value returned to false
, the while
loop will be executed once again. This will continue until there’s no more swapping, and the value of sorted
becomes true
.
Let’s test the code with a random array:
let randomArr = [9, 8, 3, 6, 1];
let sortedArr = bubbleSort(randomArr);
console.log(sortedArr); // [1, 3, 6, 8, 9]
Feel free to test it on your own next.
Bubble Sort performance notes
As you’ve seen in the implementation above, Bubble Sort always do two things for each step:
- Compare left and right values. Always gets executed.
- Swap the values. This is optional, but in the worst-case scenario, it will swap for each value.
Bubble Sort is one of the most common topics when discussing algorithms. It’s also not recommended to sort a large amount of data using Bubble Sort because its measured time complexity is O(n²)
.
When using Bubble Sort, the number of maximum steps the algorithm may take to complete the task equals to n
to the power of 2
. As the amount of data increases, the number of steps required to perform the algorithm will increase dramatically.
For an array of 5
elements, the maximum steps Bubble Sort may take to sort it is 5*5=25
Still, Bubble Sort is like a classic book in the world of Computer Science, where everyone is required to learn about it. You may also find the topic as an interview question for some software engineering jobs.