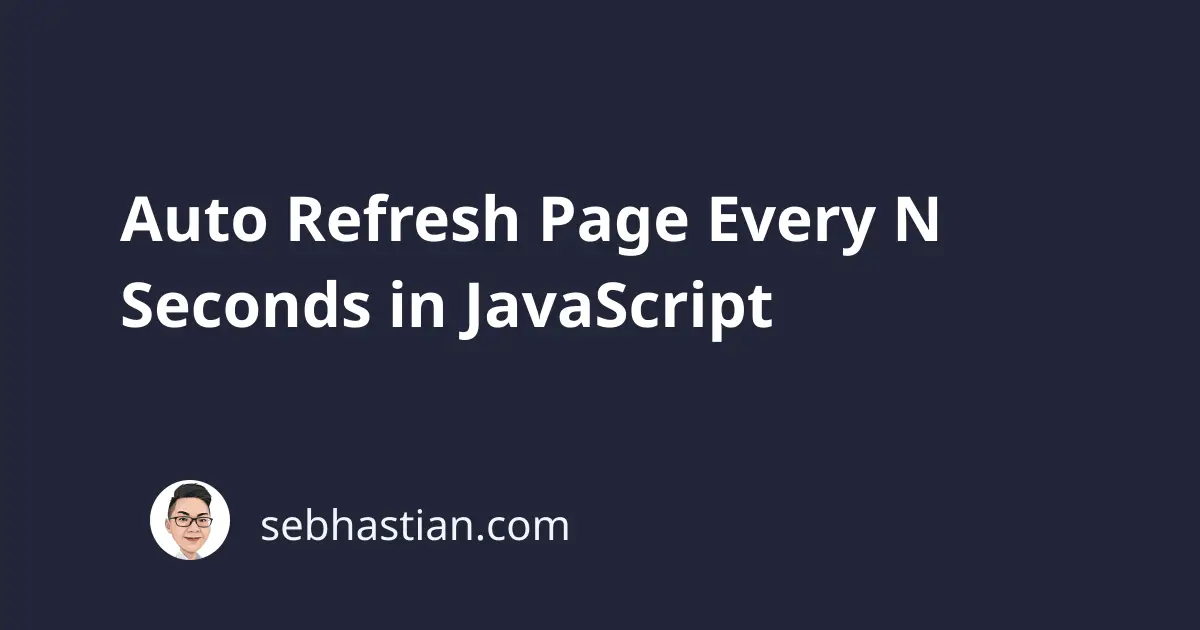
Hey there! Today we’re going to explore how to refresh a web page after a specific amount of time has passed using JavaScript.
To refresh a page every N seconds, we need to use the setTimeout()
and the location.reload()
method available from the global window
object.
The setTimeout()
function is used to execute a specific function after a certain amount of time has passed. This function accepts 2 parameters:
- The function to run once the time has passed
- The time delay before the function is executed (in milliseconds)
The following example shows how to refresh the page every 5 seconds. I will explain the code below:
<!DOCTYPE html>
<html lang="en">
<body>
<h2>Refresh Every 5 Seconds</h2>
<script>
setTimeout(() => {
window.location.reload();
}, 5000);
</script>
</body>
</html>
Inside the <script>
tag of the code above, you can see that the setTimeout()
function is called with two parameters.
First, we passed the function that will be executed after a delay. Since we want to refresh the page, we need to pass a call to the window.location.reload()
method.
Second, we passed the amount of time to delay calling the reload()
method in milliseconds. We want the delay to be 5 seconds, so we need to pass 5000 milliseconds.
The setTimeout()
method will run again after the page has been refreshed. This way, we will refresh the page every 5 seconds infinitely.
You can adjust the second parameter to delay a specific amount of time you want.
Conclusion
Now you’ve learned how to refresh a page every N seconds using JavaScript.
If you want to learn more about JavaScript, I have a JavaScript Course for Beginners that will teach you the fundamentals of JavaScript in a fun and engaging way.
See you in other articles! 👋