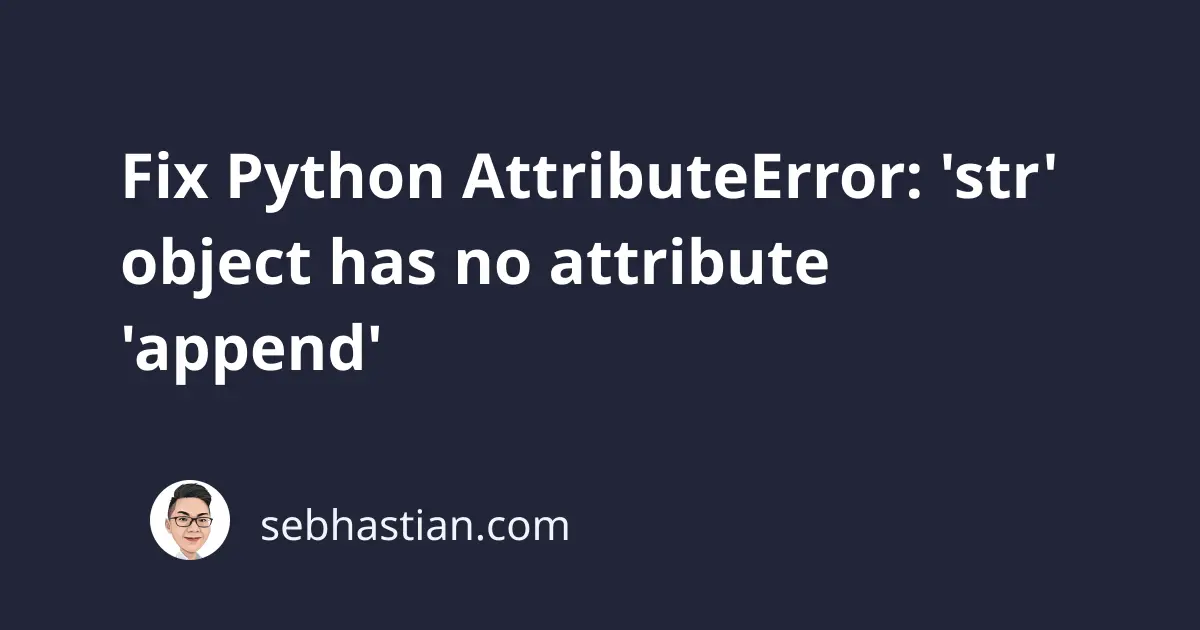
Python shows AttributeError: 'str' object has no attribute 'append'
error when you call the append()
method on a string object.
To fix this error, call the append()
method from a list and use the addition operator +
when adding characters to a string.
Suppose you try to add a string to another string using append()
as follows:
word = "Hello"
word.append(" World!")
Since the string class str
doesn’t implement the append()
method, Python responds with the following error:
Traceback (most recent call last):
File ...
word.append(" World!")
AttributeError: 'str' object has no attribute 'append'
To fix this error, you can use string concatenation to add characters to a string.
For example, you can use the +
operator to join two strings together like this:
string = "Hello, "
string += "world!"
print(string) # "Hello, world!"
Alternatively, you can use the string formatting method to add characters to a string.
The format()
method allows you to insert values into a string using placeholders as shown below:
string = "Hello, {}!"
string = string.format("world")
print(string) # "Hello, world!"
The append()
method can be used when your variable is a list instead of a string:
my_list = ["Hello"]
my_list.append("World")
print(my_list) # ['Hello', 'World']
You can also create a string from a list by using the join()
method.
The string join()
method takes an iterable (such as a list or tuple) of strings and concatenates them into a single string.
Here’s an example:
# create a list of 3 items
strings = ["Good", "morning", "love"]
# join the elements as one string
greet = " ".join(strings)
print(greet) # Good morning love
When you need to add multiple elements in a sequence, it is often more efficient to use a list instead because of the append()
method.
Once you’ve added all the elements, you can use the join()
method to concatenate them into a single string:
words = []
words.append("Hello")
words.append("world")
result = " ".join(strings)
print(string) # "Hello world"
You can choose the appropriate solution according to the needs of your program.
Conclusion
The Python error attributeError: 'str' object has no attribute 'append'
occurs when you call the append()
method on a string object.
To fix this error, you can use string concatenation, formatting, or the join()
method to add characters to a string.
If you need to add multiple elements in a sequence, using a list can help because you can use the append()
method to add the elements sequentially.