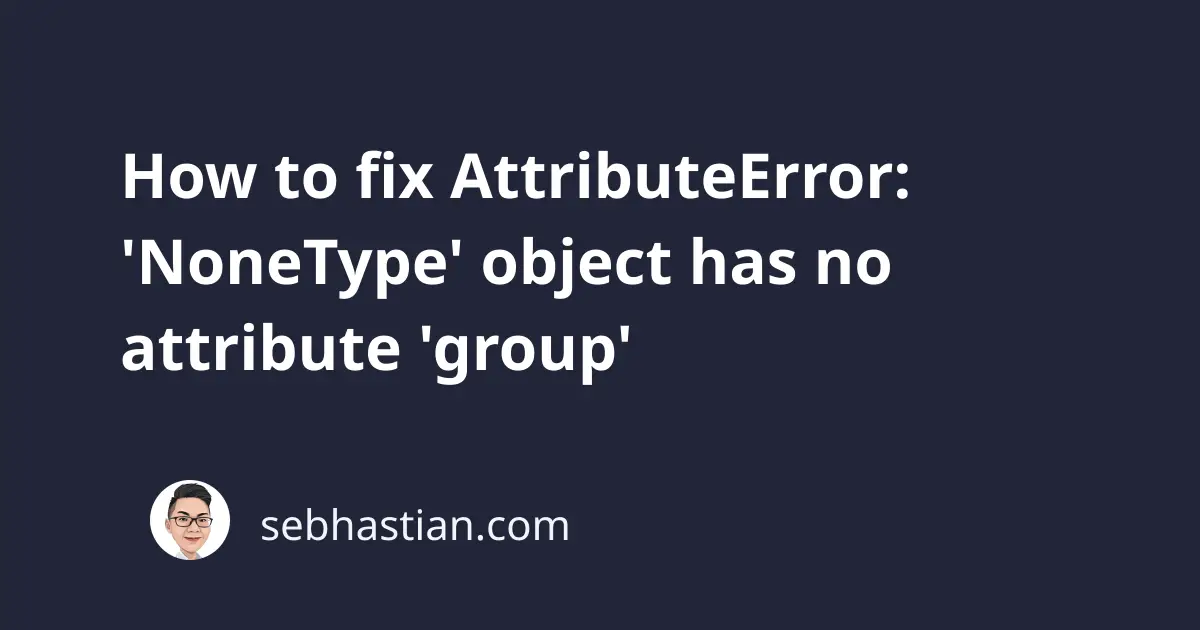
When using the re
module in Python source code, you might see the following error:
AttributeError: 'NoneType' object has no attribute 'group'
This error commonly occurs when you call the group()
method after running a regular expression matching operation using the re
module.
This tutorial shows an example that causes this error and how to fix it.
How to reproduce this error
The error comes when you try to call the group()
method on a None
object as follows:
None.group()
Output:
Traceback (most recent call last):
File "main.py", line 1, in <module>
None.group()
AttributeError: 'NoneType' object has no attribute 'group'
The reason why this error occurs when you use the re
module is that many functions in the module return None
when your string doesn’t match the pattern.
Suppose you have a regex operation using re.match()
as follows:
import re
result = re.match(r'(\w+)=(\d+)', "page:10")
print(result) # None
Because the match()
method doesn’t find a matching pattern in the string, it returns None
.
This means when you chain the group()
method to the match()
method, you effectively call the group()
method on a None
object.
re
modules like match()
, search()
, and fullmatch()
all return None
when the string doesn’t match the pattern.
How to fix this error
To resolve this error, you need to make sure that you’re not calling the group()
method on a None
object.
For example, you can use an if
statement to check if the match()
function doesn’t return a None
object:
import re
# Run a regular expression match
result = re.match(r'(\w+)=(\w+)', "a=z")
# Check if result is not None
if result is not None:
result = result.group()
print(result) # a=z
You can also include an else
statement to run some code when there’s no match:
import re
result = re.match(r'(\w+)=(\w+)', "a=z")
if result is not None:
result = result.group()
print(result)
else:
print("No match found!")
Or you can also use a try-except
statement as follows:
import re
text = "abc"
try:
result = re.match(r'(\w+)=(\w+)', text).group()
except AttributeError:
result = re.match(r'(\w+)=(\w+)', text)
print(result)
Using a try-except
requires you to repeat the call to your re
module function.
This is a bit repetitive, so you might want to use the if-else
statement which is more readable and clear.
Conclusion
The AttributeError: 'NoneType' object has no attribute 'group'
occurs when you call the group()
method on a None
object.
This error usually happens when you use the re
module in your source code. The match object in this module has the group()
method, but you might also get a None
object.
To resolve this error, you need to call the group()
method only when the regex operation doesn’t return None
.
I hope this tutorial helps. Until next time! 👋