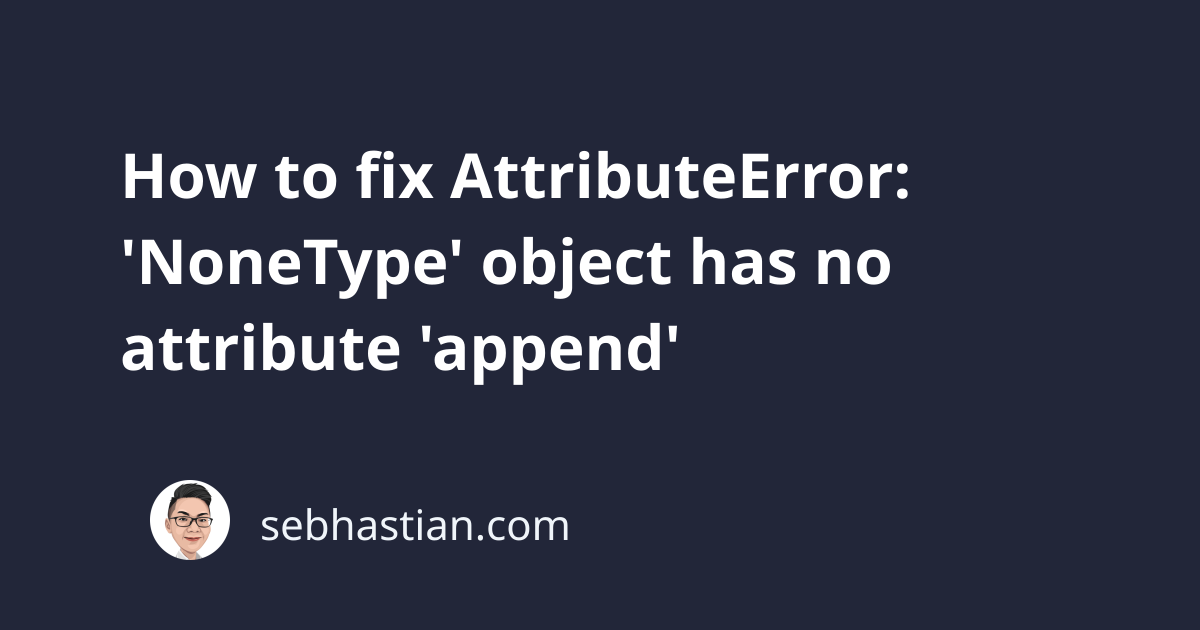
When you’re working with lists in Python, you might get the following error:
AttributeError: 'NoneType' object has no attribute 'append'
This error occurs when you call the append()
method on a NoneType
object in Python.
This tutorial shows examples that cause this error and how to fix it.
1. calling the append()
method on a NoneType object
To show you how this error happens, suppose you try to call the append()
method on a NoneType
object as follows:
fruit_list = None
fruit_list.append("Apple")
In the above example, the fruit_list
variable is assigned the None
value, which is an instance of the NoneType
object.
When you call the append()
method on the object, the error gets raised:
Traceback (most recent call last):
File "main.py", line 3, in <module>
fruit_list.append("Apple")
AttributeError: 'NoneType' object has no attribute 'append'
To fix this error, you need to call the append()
method from a list object.
Assign an empty list []
to the fruit_list
variable as follows:
fruit_list = []
fruit_list.append("Apple")
print(fruit_list) # ['Apple']
This time, the code is valid and the error has been fixed.
2. Assigning append()
to the list variable
But suppose you’re not calling append()
on a NoneType
object. Let’s say you’re extracting a list of first names from a dictionary object as follows:
customers = [
{"first_name": "Lisa", "last_name": "Smith"},
{"first_name": "John", "last_name": "Doe"},
{"first_name": "Andy", "last_name": "Rock"},
]
first_names = []
for item in customers:
first_names = first_names.append(item['first_name'])
At first glance, this example looks valid because the append()
method is called on a list.
But instead, an error happens as follows:
Traceback (most recent call last):
File "main.py", line 11, in <module>
fruit_list = fruit_list.append(item['first_name'])
AttributeError: 'NoneType' object has no attribute 'append'
This is because the append()
method returns None
, so when you do an assignment inside the for
loop like this:
first_names = first_names.append(item['first_name'])
The first_names
variable becomes a None
object, causing the error on the next iteration of the for
loop.
You can verify this by printing the first_names
variable as follows:
for item in customers:
first_names = first_names.append(item['first_name'])
print(first_names)
The for
loop will run once before raising the error as follows:
None
Traceback (most recent call last):
As you can see, first_names
returns None
and then raises the error on the second iteration.
To resolve this error, you need to remove the assignment line and just call the append()
method:
customers = [
{"first_name": "Lisa", "last_name": "Smith"},
{"first_name": "John", "last_name": "Doe"},
{"first_name": "Andy", "last_name": "Rock"},
]
first_names = []
for item in customers:
first_names.append(item["first_name"])
print(first_names)
Output:
['Lisa', 'John', 'Andy']
Notice that you receive no error this time.
Conclusion
The error “NoneType object has no attribute append” occurs when you try to call the append()
method from a NoneType
object. To resolve this error, make sure you’re not calling append()
from a NoneType
object.
Unlike the append()
method in other programming languages, the method in Python actually changes the original list object without returning anything.
Python implicitly returns None
when a method returns nothing, so that value gets assigned to the list if you assign the append()
result to a variable.
If you’re calling append()
inside a for
loop, you need to call the method without assigning the return value to the list.
Now you’ve learned how to resolve this error. Happy coding! 😃