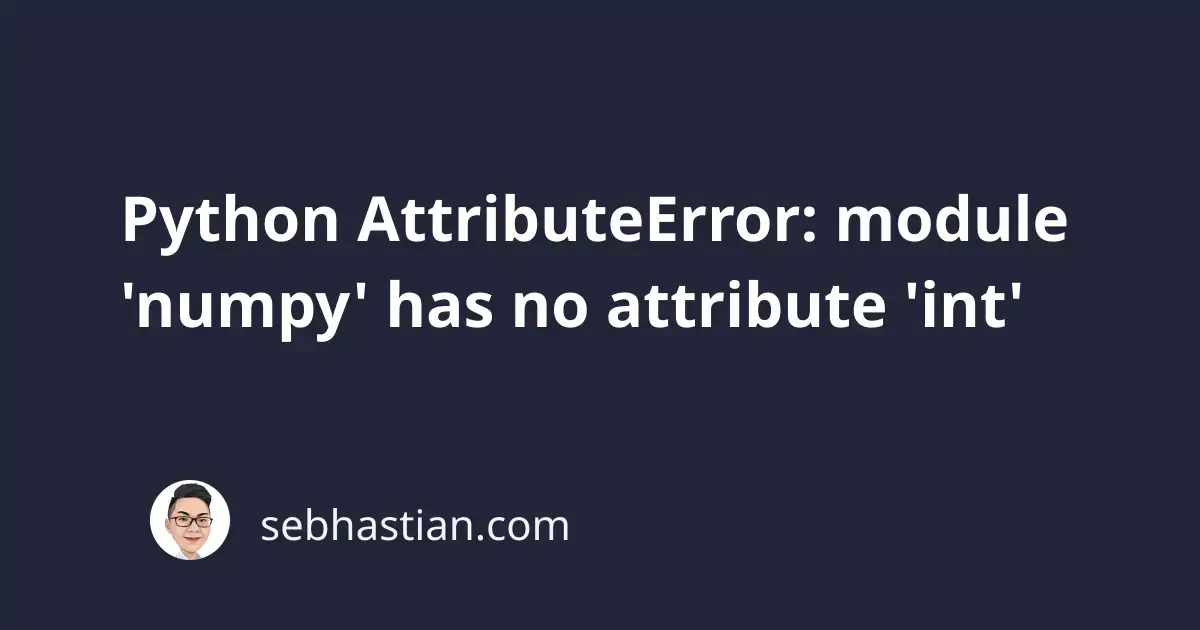
If you use the NumPy library in your Python code, there’s a chance you get the following error:
AttributeError: module 'numpy' has no attribute 'int'
AttributeError: module 'numpy' has no attribute 'object'
AttributeError: module 'numpy' has no attribute 'float'
All the errors above are similar, and this tutorial will explain why this error occurs along with simple steps you can take to fix it.
The cause: Many NumPy attributes were deprecated in the latest version
In NumPy version 1.24.0, the methods such as int()
, object()
, and float()
were deprecated and removed.
This is because they are aliases of built-in Python types which don’t exist when NumPy was first released. Nowadays, their existences are redundant and confusing to newcomers.
In the latest version of NumPy, the following attributes have been deprecated:
np.object
np.bool
np.float
np.complex
np.str
- and
np.int
If you use any one of these methods in your code as follows:
import numpy as np
score = np.int(20) # ❌
Then you’ll get the AttributeError: module 'numpy' has no attribute
response.
How to fix this error
To fix this, you need to replace the attributes above with Python built-in types or NumPy scalar types.
The following table shows you how to handle the deprecated types:
Deprecated | Python types | NumPy scalar types for precision |
---|---|---|
numpy.bool | bool | numpy.bool_ |
numpy.int | int | numpy.int_ (default), numpy.int64 , or numpy.int32 |
numpy.float | float | numpy.float64 , numpy.float_ , numpy.double (equivalent) |
numpy.complex | complex | numpy.complex128 , numpy.complex_ , numpy.cdouble (equivalent) |
numpy.object | object | numpy.object_ |
numpy.str | str | numpy.str_ |
numpy.long | int | numpy.int_ (C long), numpy.longlong (largest integer type) |
numpy.unicode | str | numpy.unicode_ |
For more information, you can see the release notes of NumPy v1.20.0.
Alright! Now let’s fix this error in our code by replacing numpy.int
with int
:
import numpy as np
score = int(20) # ✅
If you still need to use the old aliases, then you need to downgrade your NumPy version to v1.23.5 or below.
By removing the aliases for Python built-in types, the NumPy library becomes more clean and efficient.
Now, newcomers can start using NumPy with the basic Python types. They will use Numpy scalars only when they need more control over how the data is stored and represented.
Now you’ve learned how to fix the AttributeError: module 'numpy' has no attribute 'int'
which was caused by NumPy’s latest version.
I hope this tutorial is helpful. See you again! 👋