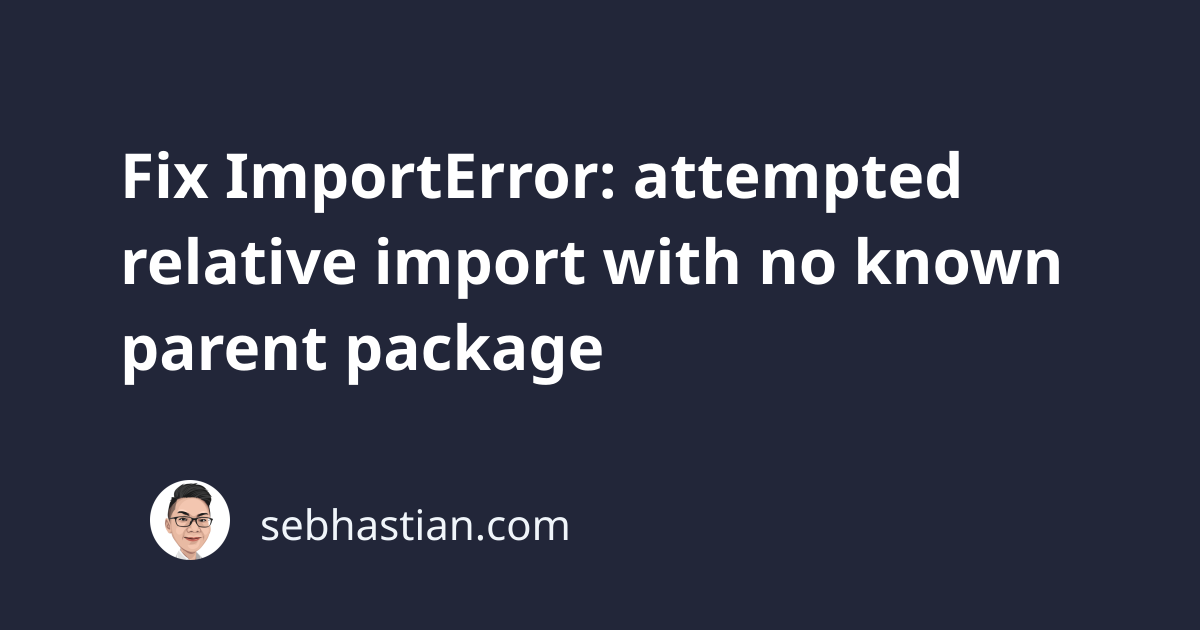
One error you might encounter when working with a Python project is:
ImportError: attempted relative import with no known parent package
This error usually occurs when you try to import a module in Python using the relative import path (using a leading dot .
or ..
)
This tutorial shows an example that causes this error and how to fix it in practice.
How to reproduce this error
First, suppose you have a Python project with the following file structure:
.
└── project/
├── helper.py
└── main.py
The helper.py
script contains a function as follows:
def greet():
print("Hello World!")
Next, you tried to import helper.py
into main.py
as follows:
from .helper import greet
print("Starting..")
greet()
The output will be:
Traceback (most recent call last):
File "main.py", line 1, in <module>
from .helper import greet
ImportError: attempted relative import with no known parent package
This error occurs because we used a relative import, which doesn’t work when you’re importing a module in the same directory as the main script you executed using Python.
Python doesn’t consider the current working directory to be a package, so you can’t do a relative import unless you run your main.py
script from the parent directory.
How to fix this error
To resolve this error, you need to change the relative import to an absolute import by removing the leading dot:
from helper import greet
print("Starting..")
greet()
The output will be:
Starting..
Hello World!
Python is able to find the helper
module, so we didn’t get any errors this time.
If you still want to use the relative import path, then you need to run the main script as a module from its parent directory.
Since the main.py
file is located inside the project
directory, you can run the script with this command:
python -m project.main
# or
python3 -m project.main
This time, the relative import works because Python considers the project
directory to be a package. It keeps track of what’s inside the directory.
If you run the script directly without specifying the parent directory as follows:
python3 -m main
Then the relative import error will occur again.
The same solution also works when you try to do sibling imports. Suppose you have the following file structure in your project:
.
└── project/
├── lib
│ └── helper.py
└── src
└── main.py
And you try to import the helper.py
script into the main.py
script as follows:
from ..lib.helper import greet
print("Starting...")
greet()
To make the relative import successful, you need to run the main
script from the project
directory like this:
python -m project.src.main
# or
python3 -m project.src.main
If you run the main.py
script directly, you’ll get the same error:
$ python3 main.py
Traceback (most recent call last):
File "/main.py", line 1, in <module>
from ..lib.helper import greet
ImportError: attempted relative import with no known parent package
The internal workings of Python doesn’t allow you to do relative import unless you specify the top-level directory where all your modules are located.
By adding the root directory of the project when running the main script, you can solve the relative import error.
I hope this tutorial is helpful. Happy coding! 👍