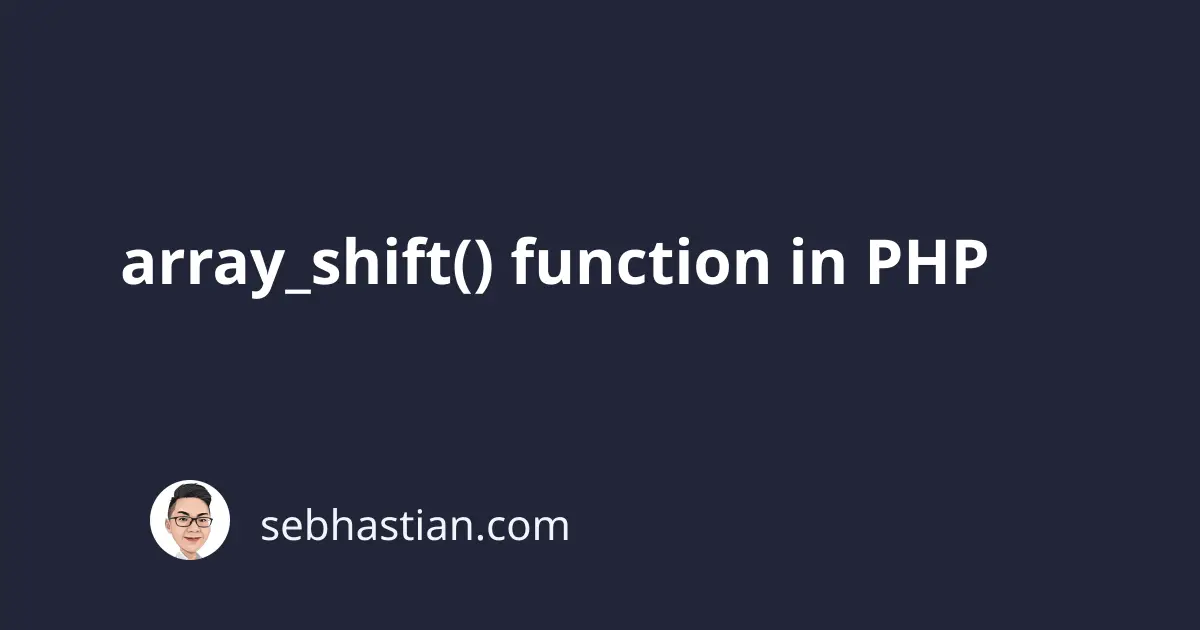
The array_shift()
function in PHP is used to shift the first element of an array.
This function removes the first element of an array and returns it, shortening the array by one element and moving the index of the remaining elements down.
Here’s the function syntax:
array_shift(array &$array): mixed
The array_shift
function accepts an array as its parameter.
The return value of the function is the same as the shifted element’s type.
Here are some examples of calling the array_shift()
function:
$arr = ["Cat", "Tiger", "Bear"];
$element = array_shift($arr);
echo $element; // Cat
print_r($arr);
// Array
// (
// [0] => Tiger
// [1] => Bear
// )
As you can see, the first element of the $arr
variable (“Cat”) is removed from the array and returned with the array_shift()
call.
The array is modified, moving the “Tiger” and “Bear” elements one index down.
This function also works for a numbers array:
$arr = [7, 8, 9, 10];
$element = array_shift($arr);
echo $element; // 7
print_r($arr);
// Array
// (
// [0] => 8
// [1] => 9
// [2] => 10
// )
array_shift()
will also work when you have an associative array.
When the keys are numeric, array_shift()
will assign new keys starting from 0.
But when you have string keys, then there’s no change at all:
// 👇 1) associative array with string keys
$arr = ["A" => "Orange", "D" => "Apple", "Z" => "Banana"];
$element = array_shift($arr);
echo $element; // Orange
print_r($arr);
// Array
// (
// [D] => Apple
// [Z] => Banana
// )
// 👇 2) associative array with number keys
$arr = [0 => "Orange", 3 => "Apple", 101 => "Banana"];
$element = array_shift($arr);
echo $element; // Orange
print_r($arr);
// Array
// (
// [0] => Apple
// [1] => Banana
// )
As you can see, the associative array keys are reordered in the second example, but left alone in the first example.
The array_shift()
function is useful when you need to remove the first element in a PHP array.