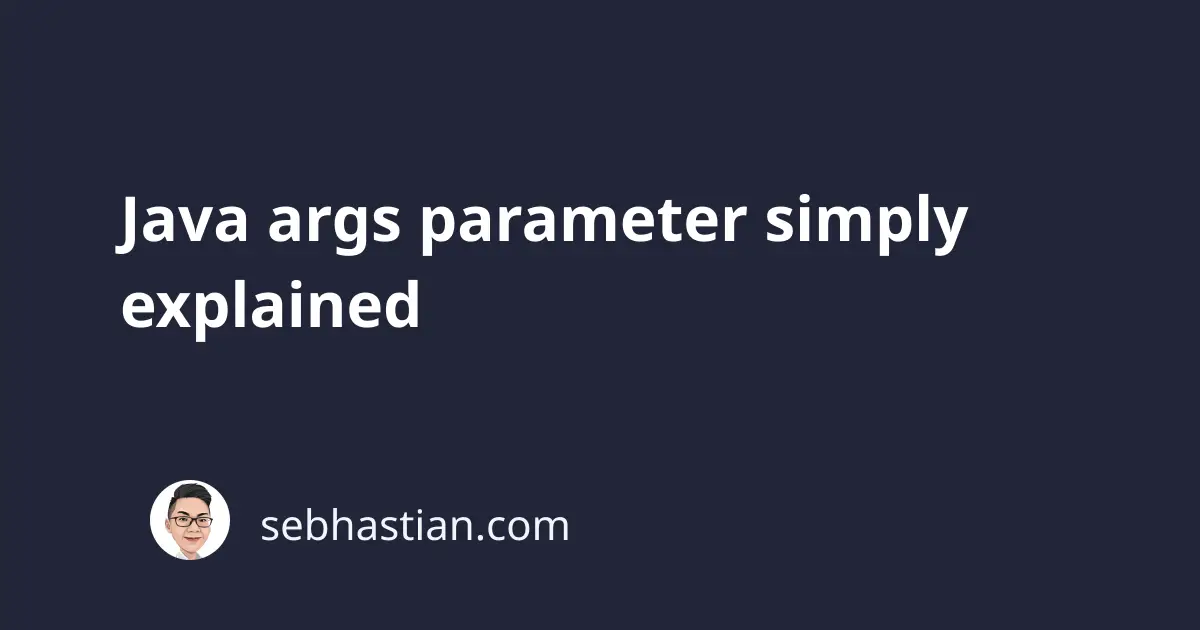
When inspecting Java code from tutorials or open-source projects, you may see a main()
method that has an args
parameter.
The args
parameter is defined as an array of String
values as shown below:
class Main {
public static void main(String[] args) {
// your code here...
}
}
The args
parameter stands for arguments, and they contain the arguments you specify when running the code from the command line.
To see an example, suppose you add a for
loop to print the values in the args
parameter as follows:
class Main {
public static void main(String[] args) {
for (String str: args){
System.out.println(str);
}
}
}
The arguments are passed when you run the Java class using the java
command-line program.
You only need to specify the arguments next to the Java class name like this:
> java Main.java nathan sebhastian
args output:
nathan
sebhastian
As you can see, the strings nathan
and sebhastian
passed as the argument to the Main.java
class main()
method.
The name args
is the default convention in Java. You are free to rename the parameter if you want to.
When you’re running a Java project from IDEs like Android Studio and IntelliJ IDEA, you can create a Run Configuration that includes your arguments.
Open your Java class using the IDE, then right-click anywhere on the editor pane and select the Modify Run Configuration… option:
In the Run Configuration window, add your arguments to the Program arguments option.
For more than one argument, add a space between the arguments. In the example below, three arguments are added to the Run Configuration:
When you’re ready, click the OK and run the main()
method from the IDE.
You’ll see the arguments printed out on the console as follows:
args output:
nathan
sebhastian
programmer
Process finished with exit code 0
And that’s how the args
parameter for Java main()
method works.
You can add as many arguments as you need and they will all be stored inside the args
parameter.