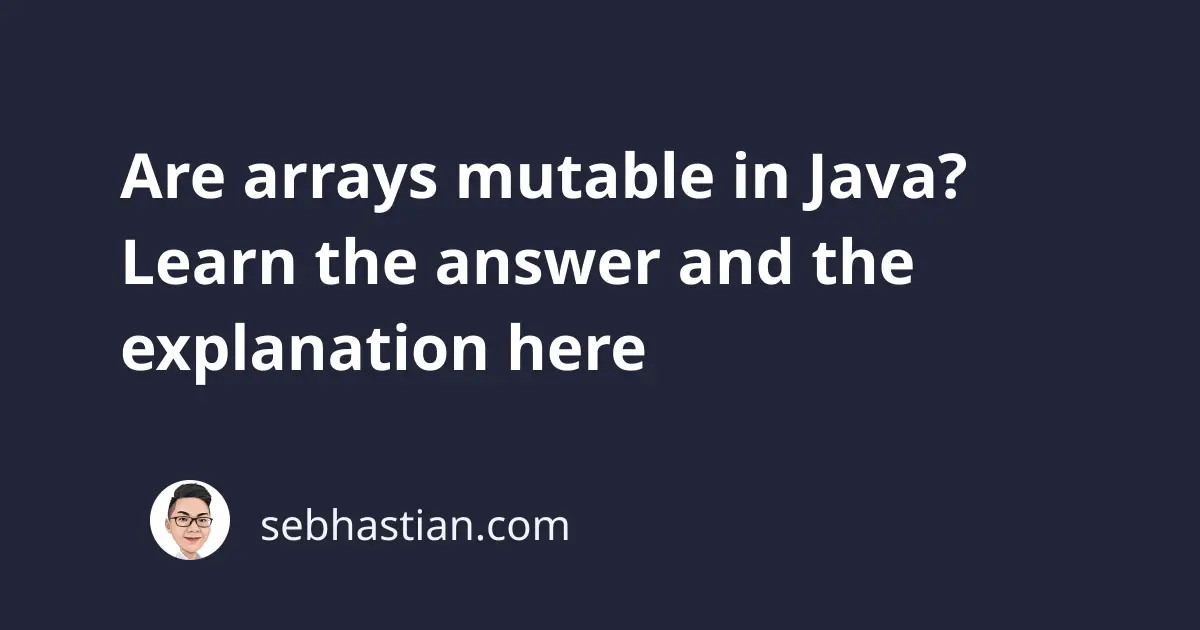
In programming, mutability is the ability to change the state of an object after it has been created.
A Mutable object means the object state or values can be changed, while an immutable object means the object values are fixed.
Arrays in Java are mutable because you can still change the values of the array after it has been created.
In the following example, The value of myArray
at index 2
are changed after it has been initialized:
Integer[] myArr = {1, 2, 3};
myArr[2] = 55;
System.out.println(Arrays.toString(myArr));
The output of the println()
method above will show [1, 2, 55]
instead of [1, 2, 3]
.
But even though a Java array is mutable, the type and length of the array are final on initialization.
This means once you create an Integer
array of 3
elements, that element can only hold 3
elements of the Integer
type for its lifetime.
The same goes when you create an array of String
type:
String[] myArr = {"Java", "Kotlin"};
myArr[0] = 55; // ERROR: Required String
Mutability is only concerned with whether or not you can change the value of the object.
This is why an array is mutable even though you can’t change the type and length of the array after initialization.
Are arrays mutable in Java? The answer is yes.