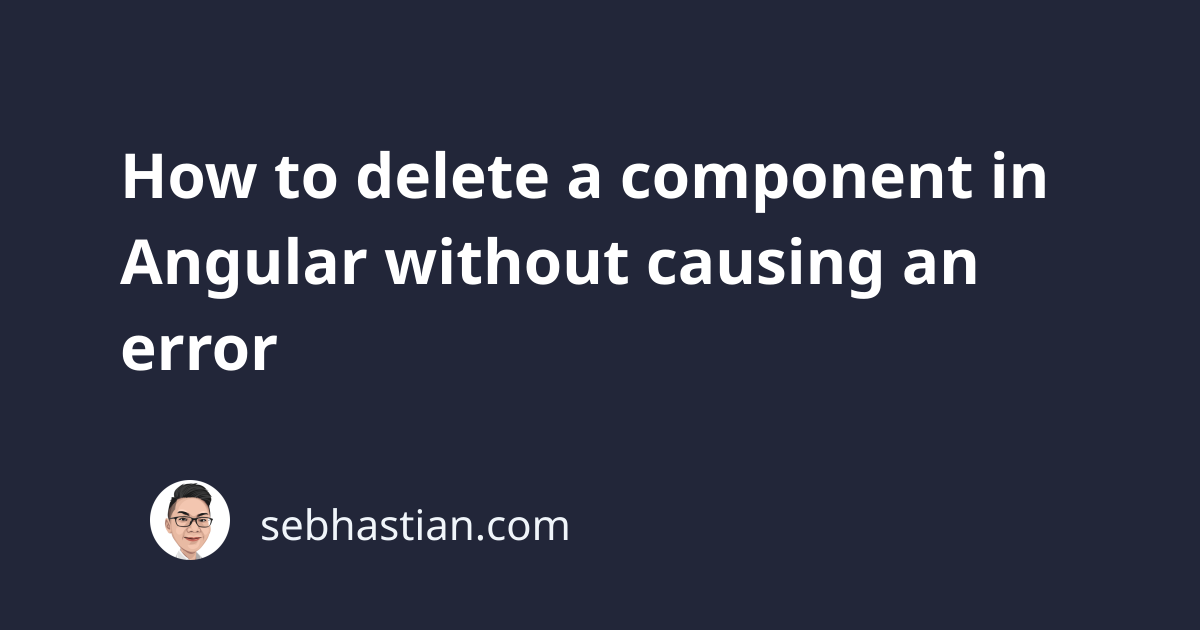
There’s no command to delete a component with the Angular CLI tool, so you need to do it manually.
Here are the steps to delete an Angular component:
- Remove the
import
that refers to the component fromapp.module.ts
file - Also remove the component from the
declarations
array in your@NgModule
decorator - Delete the component folder from your project
- Find and delete all references to the component using the Find command
Let’s see an example of deleting an Angular component. Suppose you want to delete a component named User
that you’ve created previously.
First, open the app.module.ts
file, then delete references to the component as shown below:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { UserComponent } from './user/user.component';
@NgModule({
declarations: [
AppComponent,
UserComponent
],
imports: [
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Delete references to the component in the highlighted import
and @NgModule
lines as shown above.
Once done, save the changes and delete the component folder:
By default, the component folder should follow the name of the component, so UserComponent
should be in the user
folder.
The next step is to find all references to the component in your project and delete them all.
If you’re using Visual Studio Code, then you can run the Find command with CTRL + SHIFT + F
(Windows and Linux) or CMD + SHIFT + F
(macOS)
Type your component’s name in the search field, then see if there are existing references to that component:
Remove any found references until nothing is found, and you should be able to run the application without facing any errors.
Now you’ve learned how to delete a component in an Angular application. Nice work! 👍