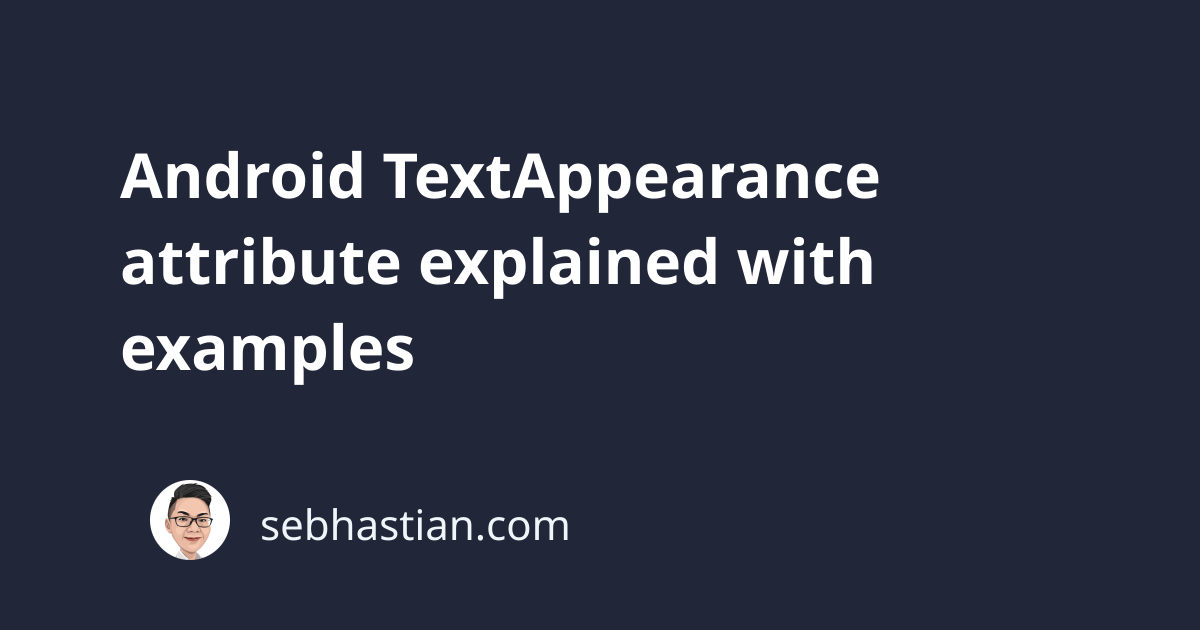
The Android TextAppearance
attribute is a special attribute used to apply text-specific styling to your View
components.
The TextAppearance
attribute is created to help Android developers create a modular styling system for their applications.
A single Android View
component can have one style
attribute applied to it. You can’t apply two styles as shown below:
<TextView
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:text="Hello World!"
style="@style/PaddingSmall"
style="@style/TextAppearance.Important"
/>
The TextAppearance
attribute is used to overcome the above limitation.
Here’s an example of how to define a TextAppearance
attribute:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<style name="TextAppearance.Important" parent="">
<item name="android:textSize">28sp</item>
<item name="android:color">@color/black</item>
</style>
</resources>
The TextAppearance
style above can be applied to a view using the android:textAppearance
attribute.
Here’s an example of applying the style to a TextView
component:
<TextView
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:text="Hello World!"
android:textAppearance="@style/TextAppearance.Important"
/>
With the android:textAppearance
attribute set, Android will apply the styles to the component.
The TextAppearance
attribute works just like the style
attribute, but it can only apply the following list of attributes:
textSize
typeface
textStyle
textColor
textColorHighlight
textColorHint
textColorLink
shadowColor
shadowDx
shadowDy
shadowRadius
textAllCaps
fontFamily
elegantTextHeight
letterSpacing
fontFeatureSettings
fontVariationSettings
fallbackLineSpacing
textFontWeight
textLocale
lineBreakStyle
lineBreakWordStyle
You can add a styling attribute that’s not supported by TextAppearance
, but that style won’t be applied to the component.
For example, suppose you add a layout_width
attribute to the previous TextAppearance
style:
<style name="TextAppearance.Important" parent="">
<item name="android:textSize">28sp</item>
<item name="android:color">@color/black</item>
<item name="android:layout_width">match_parent</item>
</style>
There will be no error in the XML resource file, but the attribute will still be ignored.
when you remove the android:layout_width
attribute from the TextView
, Android Studio will produce the following error:
Element TextView doesn't have required attribute android:layout_width
To apply the styles unsupported by the TextAppearance
attribute, you need to apply using the style
attribute.
For example, here’s a PaddingSmall
style created in the XML resource file:
<style name="PaddingSmall">
<item name="android:padding">8dp</item>
</style>
You can apply the style to your components like this:
<TextView
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:text="Hello World!"
style="@style/PaddingSmall"
android:textAppearance="@style/TextAppearance.Important"
/>
By using the TextAppearance
attribute, you can define text-specific stylings separated from the regular style, allowing you to apply two different style rules to your view components.
Android styling hierarchy and TextAppearance
Please note that any same style you add to the View
definition itself will override the styles defined in the TextAppearance
attribute.
Based on their importance, here’s how Android styling works:
- Programatically applied attributes
- Attributes applied to the view
- Applying a specific style
- The default style for the view
- A universal theme for your application
- View-specific styling such as
TextAppearance
As you can see, the TextAppearance
attribute is given the lowest priority. Keep the hierarchy in mind as you style your components.
When you don’t see any change in the component’s appearance, then most likely you have other rules applied to the view that overrides the TextAppearance
styles.
And that’s how the TextAppearance
attribute works in Android. 👌
For more information, see the Android TextAppearance
explanation