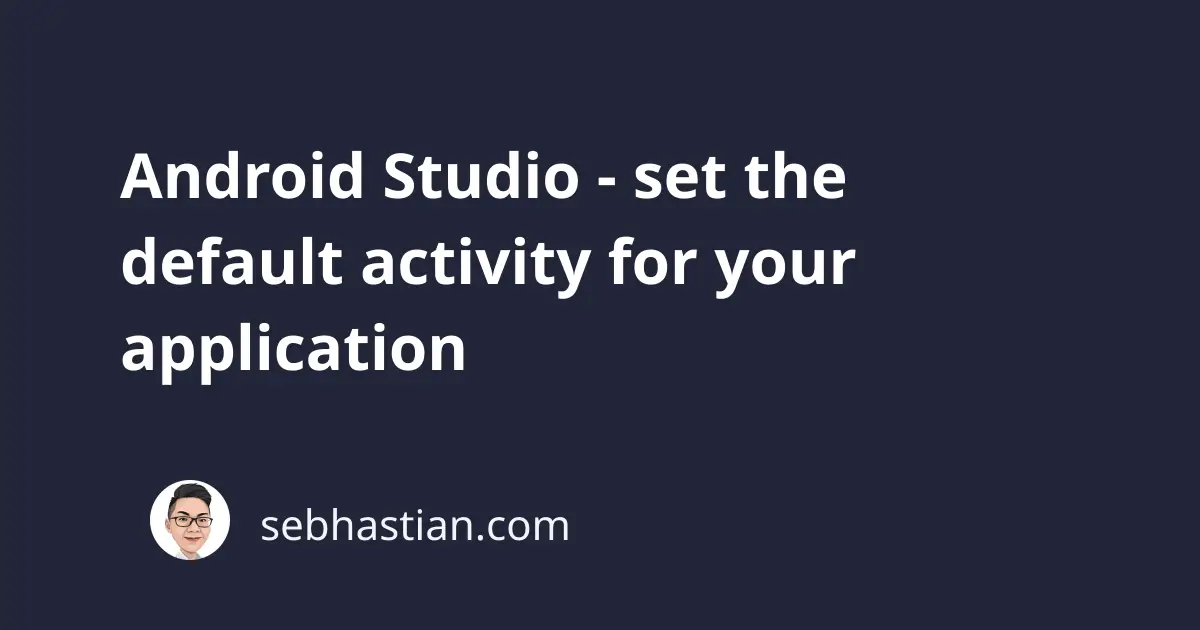
The Android system finds the default activity to launch by looking at your AndroidManifest.xml
file.
The default activity will be the activity that has an <intent-filter>
tag as follows:
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
When Android Studio generates your application, the <intent-filter>
is usually added to the MainActivity
class as shown below:
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
When you add a new activity, then that activity will be added to the manifest file as well, but without the <intent-filter>
tag.
In the example below, there’s a second activity named SplashScreen
added to the manifest:
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity
android:name=".SplashScreen"
android:exported="false" />
To set SplashScreen
activity as the default activity, you need to move the <intent-filter>
tag like this:
<activity
android:name=".MainActivity"
android:exported="true">
</activity>
<activity
android:name=".SplashScreen"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
You also need to set the exported
attribute as true
as shown above. This is a new requirement added as of Android version 12.
Once done, run the application and you should see the first activity change to the one that has the <intent-filter>
tag.
If you didn’t see the new activity launched, then you need to check the launch configuration in your Android Studio.
At the top menu in your Android Studio, select Run > Edit Configurations… menu to open the Run/Debug Configurations window.
Inside the window, make sure that the Launch option is set to Default Activity as shown below:
Click the OK button and run your application again.
This time, the default activity launched should follow the <intent-filter>
set in your AndroidManifest.xml
file.
And that’s how you set the default activity for your Android application. 😉