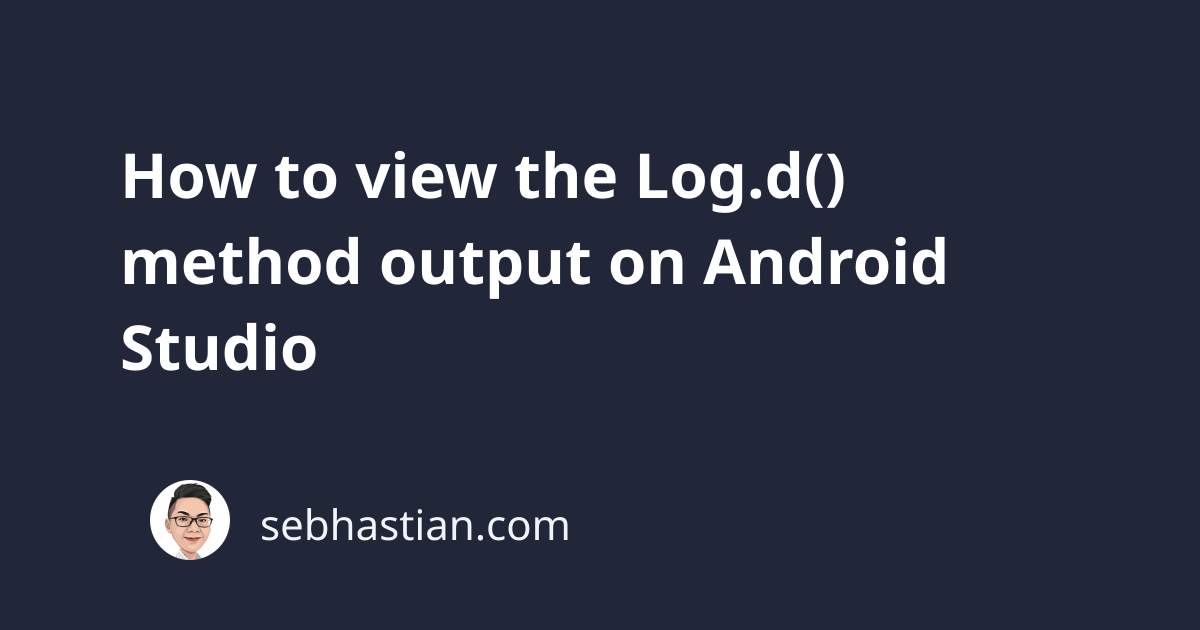
The Android Log
class is a utility class used to generate system messages.
The log messages will be captured by the Logcat tool, a program designed to capture log messages on Android devices.
The Android Studio also has a Logcat window so you don’t have to access the Logcat using the command line.
The Log.d()
method is used to display messages with the Debug level priority on the Logcat.
The method requires two arguments:
- The
tag
argument is the tag of the message - The
msg
argument is the message to display on the Logcat
Let’s see an example of the method in action. Add the following Log.d()
call in your onCreate()
method:
import android.util.Log;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Log.d("MainActivity","onCreate() method finished");
}
}
Next, you build the application and run it on your Android device.
The Log.d()
method will generate the following log message in the Logcat:
2022-05-27 16:01:45.034 7722-7722/org.metapx.myapplication
D/MainActivity: onCreate() method finished
To view the output from Android Studio, you need to open the Logcat window located near the bottom of the main window.
Change the filter to show only Debug level messages and put the LEVEL/TAG
in the filter box as shown below:
The Log.d()
method is commonly used to print out the values assigned to your variables.
For example, you can print out the response
object that you get from a Volley HTTP request as follows:
String url = "https://api.coindesk.com/v1/bpi/currentprice.json";
RequestQueue queue = Volley.newRequestQueue(this);
JsonObjectRequest stringRequest = new JsonObjectRequest(
Request.Method.GET, url, null,
response -> Log.d("MainActivity", "Response is: " + response.toString()),
error -> Log.e("MainActivity", "Request error: " + error.toString()));
queue.add(stringRequest);
The above code will send a GET
request to the public CoinDesk API.
The JSON object response
can be logged into the Logcat as shown below:
2022-05-27 16:33:51.160 11146-11146/org.metapx.myapplication
D/MainActivity: Response is:
{"time":{"updated":"May 27, 2022 09:33:00 UTC","updatedISO":"2022-05-27T09:33:00+00:00",
"updateduk":"May 27, 2022 at 10:33 BST"},"disclaimer":"This data was produced from the
CoinDesk Bitcoin Price Index (USD). Non-USD currency data converted using hourly
conversion rate from openexchangerates.org","chartName":"Bitcoin",
"bpi":{"USD":{"code":"USD","symbol":"$","rate":"28,986.2224",
"description":"United States Dollar","rate_float":28986.2224},"GBP":{"code":"GBP",
"symbol":"£","rate":"22,971.2334","description":"British Pound Sterling",
"rate_float":22971.2334},"EUR":{"code":"EUR","symbol":"€",
"rate":"27,013.5361","description":"Euro","rate_float":27013.5361}}}
The output looks confusing because it’s in JSON format. You need to copy the response string into a JSON prettier service to make it more readable.
For the tag name in your log messages, It’s recommended to declare a TAG
constant in your class as shown below:
public class MainActivity extends AppCompatActivity {
private static final String TAG = "MainActivity";
// ...
}
When you need to display messages using the Log
class, use the TAG
string for the tag argument:
JsonObjectRequest stringRequest = new JsonObjectRequest(
Request.Method.GET, url, null,
response -> Log.d(TAG, "Response is: " + response.toString()),
error -> Log.e(TAG, "Request error: " + error.toString()));
This way, you don’t need to repeatedly specify your own tag when defining printing a log message.
The Log.d()
method is available to use with both Java and Kotlin language.