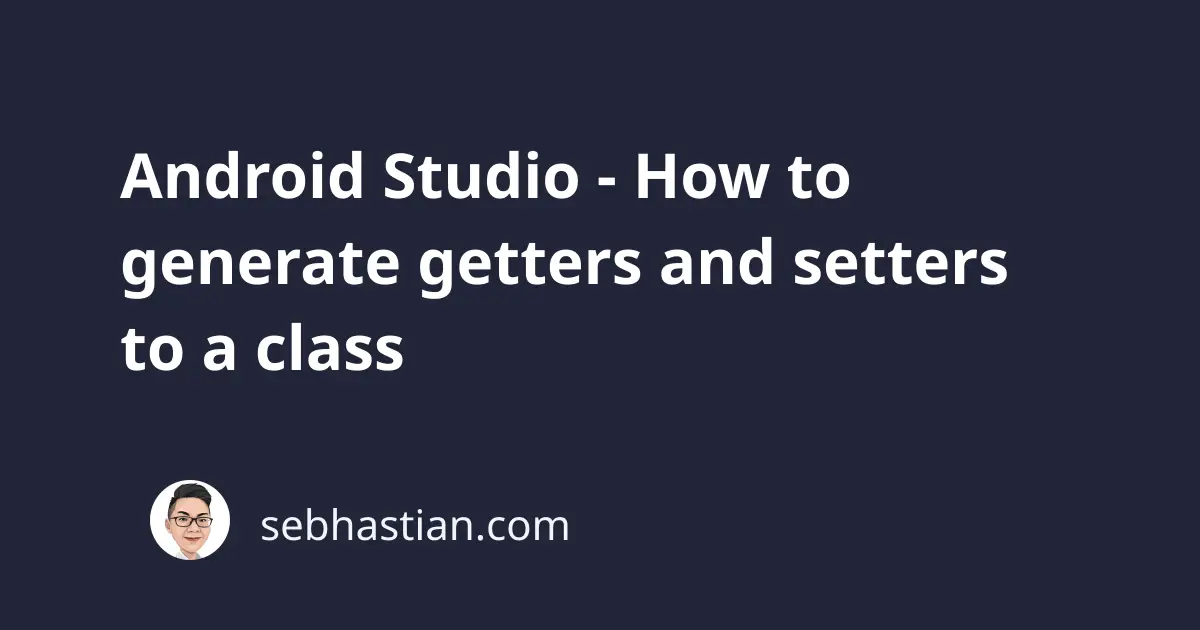
Android Studio provides you with a helper menu to generate getters and setters for class
fields.
Here’s how to generate getters and setters for Java class
fields:
- Press
command + N
for Mac - Press
Alt + Insert
for Windows and Linux.
In the following screenshot, you can see the Generate setter and getter option highlighted from the Generate menu:
You can also reach the same menu by right-clicking anywhere inside your class
in the code editor and select the Generate… option.
A context menu should appear as shown below:
Alternatively, you can also use the top bar Code menu to generate getters and setters for a class:
Code > Generate… > Getter and Setter
Inside the Getter and Setter menu, select all the fields you want to generate getters and setters methods and click OK.
You should see getters and setters generated in your code as shown below:
public class User {
private String username;
private String password;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
And that’s how you generate getters and setters for a Java class.
Generate getters and setters for a Kotlin class
By default, Kotlin automatically generate getters and setters for all your class properties.
When you define the following code in Kotlin:
class Profile {
var name: String = "Nathan"
var age: Int = 29
}
Getters and setters will be auto-generated in your class
like this:
class Person {
var name: String = "Nathan"
var age: Int = 29
// getter
get() = field
// setter
set(value) {
field = value
}
}
In Kotlin, there’s no need to define getters and setters manually to get the class
attribute or property values.
The following Java code:
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
Is equivalent to the following Kotlin code:
var name: String = ""
In Kotlin, class
fields (or properties) are always accessed through the getter and setter of that class. When you run the classname.property = value
assignment, the set()
function is called internally.
When you try to get property value using the classname.property
syntax, then the get()
function is called.
There’s no need to create private
properties with public
accessors/ mutators like in Java.
For this reason, Android Studio doesn’t provide an option to generate getters and setters for Kotlin classes.
At times, you might want to set the setters as private to prevent change outside of the class. You can do so with the following code:
class Profile {
var name: String = "Nathan"
private set
var age: Int = 29
private set
}
You need to set the access level of the setters individually for each property.
Now you’ve learned how to generate getters and setters method with the help of Android Studio. Good work! 👍