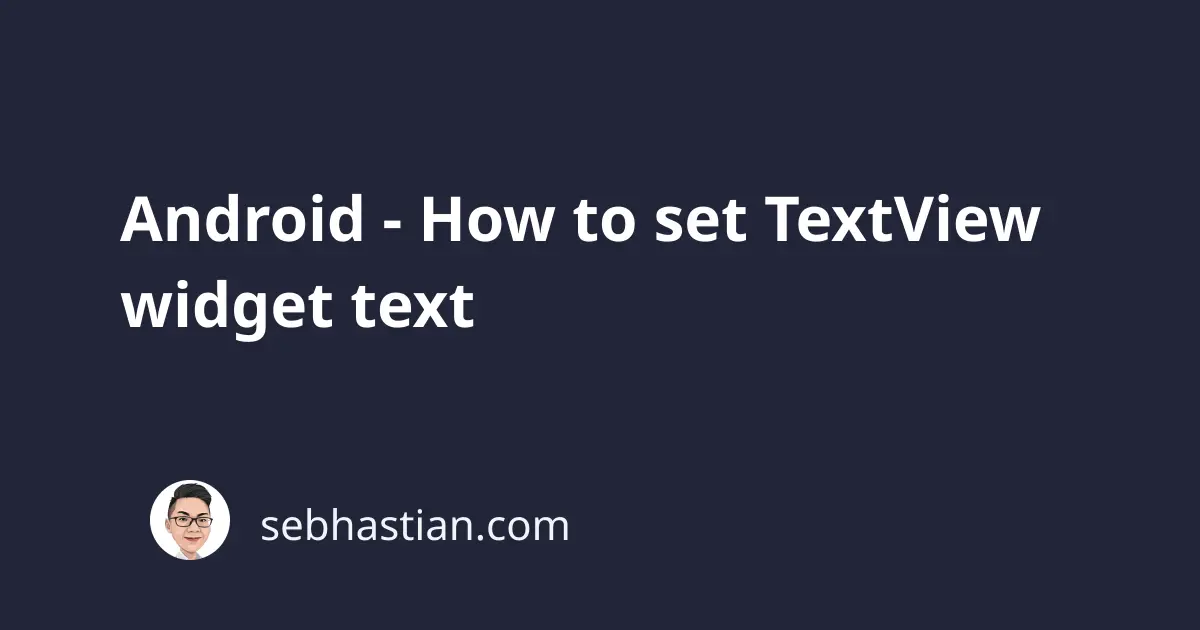
The Android TextView
widget is a UI component used for displaying text in your interface.
The text displayed in the TextView
can be set using the android:text
attribute in your XML layout file.
Here’s an example of setting the TextView
’s text value to “Good Morning!”:
<TextView
android:id="@+id/id_text_view"
android:text="Good Morning!"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
The text displayed by the TextView
will be the same as the value of the android:text
attribute.
Set the TextView text programmatically
You can also set the TextView
’s text attribute programmatically in your Activity
class.
Inside the Activity
class where you want to set the text, find the TextView
using the findViewById()
method.
Then, use the setText()
method to set the new text.
Here’s the code example in Java:
TextView myTextView = findViewById(R.id.id_text_view);
myTextView.setText("Good Evening!");
In Kotlin, you can set the text
property of the TextView
widget directly.
You don’t need to call the setText()
method as shown below:
val myTextView = findViewById<TextView>(R.id.textView)
myTextView.text = "Good Evening!"
And that’s how you set the TextView
text programmatically.
Next, let’s learn how you can use a string resource to set the TextView
text.
Using a resource string to set the TextView text
Generally, you are recommended to use the @string
resource value so that the text can be updated from one place.
Android Studio helps you to extract the string resource when you open the context action menu (ALT + ENTER
)
Suppose you have three text views in different XML files that display the “Good Morning!” text.
When you hard-code the string, then you need to change all occurrences of the “Good Morning!” text manually.
By using a string resource, you only need to change the value of the string in the strings.xml
file:
<resources>
<string name="good_morning">Good Morning!</string>
</resources>
All TextView
widgets with the @string/good_morning
text attribute will use the resource string above:
<TextView
android:id="@+id/id_text_view"
android:text="@string/good_morning"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
You can also use a string resource value when setting the text programmatically.
Here’s the code example in Java:
TextView myTextView = findViewById(R.id.id_text_view);
myTextView.setText(R.string.good_morning);
And here’s the code example in Kotlin:
val myTextView = findViewById<TextView>(R.id.textView)
myTextView.setText(R.string.good_morning)
// or
myTextView.text = getString(R.string.good_morning)
Note that you still need to use the setter method when using the string resource.
Otherwise, you need to use the getString()
method to retrieve the string value from the resource.
Now you’ve learned how to set the TextView
widget text in Android development. Nice work! 👍