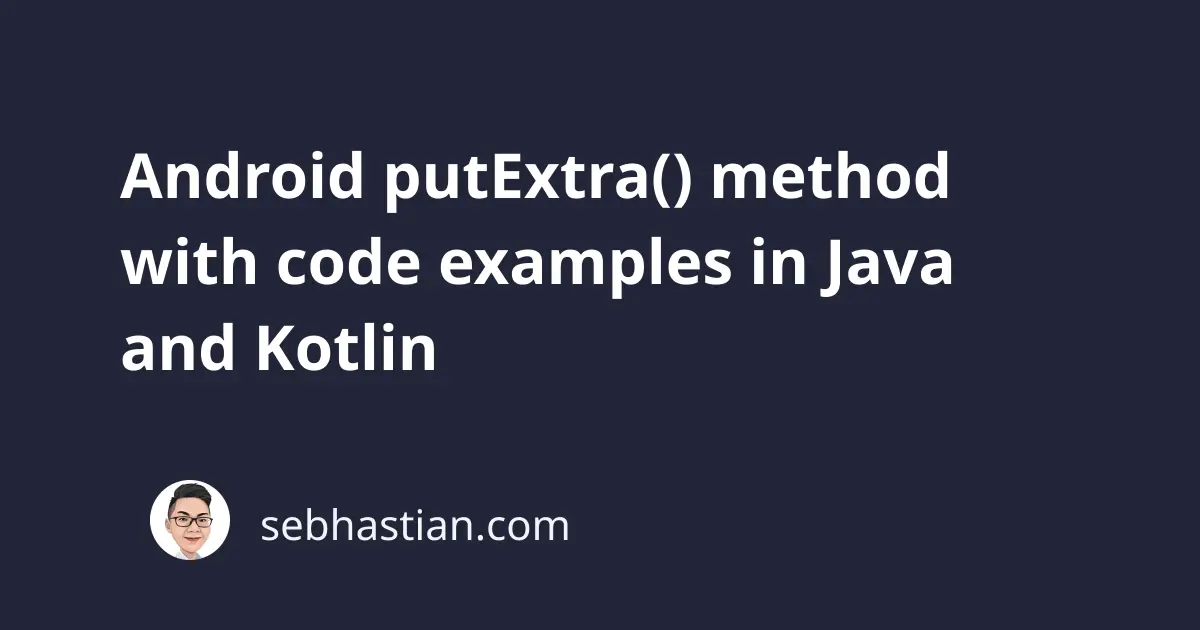
The Android Intent
class is commonly used to move from one activity to another.
The Intent
class has a method named putExtra()
that you can use to put extended data to the intent.
The main use of the putExtra()
method is to send values you need in the next activity.
The method requires two arguments:
- The
name
to identify and retrieve the value later (String
type) - The
value
you want to send, identified by thename
you define as the first argument (Any valid type)
For example, suppose you want to send a Hello World!
string from MainActivity
to SecondActivity
. This is how you do it:
// In MainActivity class
Intent secondActivityIntent = new Intent(this, SecondActivity.class);
secondActivityIntent.putExtra("message", "Hello World!");
startActivity(secondActivityIntent);
First, you create a new Intent
object. Then, you call the putExtra()
method from that object.
The first argument is the name
of the extra, which is message
in the example above.
In the SecondActivity
class, you can retrieve the extra using the getStringExtra()
method:
// In SecondActivity class
String msg = getIntent().getStringExtra("message");
Log.d("org.metapx.javaapp", msg);
The msg
variable above will contain the Hello World!
string saved under the message
name identifier.
Android has many get__Extra()
methods, and each is used to retrieve an extra of a specific type through the Intent
object.
If you send a boolean
value, you need to retrieve it using getBooleanExtra()
.
For an int
value, you need to use the getIntExtra()
, and so on.
The putExtra() method in Kotlin
The syntax for the putExtra()
method in Kotlin is as shown below:
// In MainActivity class
val secondActivityIntent = Intent(this, SecondActivity::class.java)
secondActivityIntent.putExtra("message", "Hello World!")
startActivity(secondActivityIntent)
When retrieving the extra, you can use the getExtra()
method as follows:
// In SecondActivity class
val msg = intent.getStringExtra("message")
The intent
field will call the getIntent()
method under the hood, so you don’t need to explicitly call the getIntent()
method.
Next, let’s learn how you can pass
Sending a serializable object as an extra
Sending many values using an Intent
object is a bit tedious because you need to repeatedly call the putExtra()
method for each value.
The example below shows how you send three values using the Intent
object:
secondActivityIntent.putExtra("username", "Nathan");
secondActivityIntent.putExtra("age", 23);
secondActivityIntent.putExtra("isLegal", true);
Instead of sending the extras one by one, you can create a Serializable
class that you can use to store your data in one object.
First, create a class
that implements the Serializable
interface. For our example, let’s name it Profile
:
class Profile implements Serializable {
String username;
int age;
boolean isLegal;
Profile(String username, int age, boolean isLegal) {
this.username = username;
this.age = age;
this.isLegal = isLegal;
}
}
In your MainActivity
class, create a new Profile
object with the data you want to send to the SecondActivity
.
Send the Profile
object as an extra with the putExtra()
method:
// In MainActivity class
Profile prof = new Profile("Nathan", 23, true);
secondActivityIntent.putExtra("profile", prof);
startActivity(secondActivityIntent);
Next, you need to get the profile
extra from the SecondActivity
class.
Use the getSerializableExtra()
method to get the object, then cast the object as the Profile
class type:
// In SecondActivity class
Serializable s = getIntent().getSerializableExtra("profile");
Profile p = (Profile) s;
Log.d("org.metapx.javaapp", p.username); // Nathan
Log.d("org.metapx.javaapp", String.valueOf(p.age)); // 23
Log.d("org.metapx.javaapp", String.valueOf(p.isLegal)); // true
Using a Serializable
class is a great way to send data between activities.
In Kotlin, the code is shorter since you can create a data class
of Serializable
type like this:
data class Profile(
val username: String,
val age: Int,
val isLegal: Boolean
) : Serializable
Use the data class
to create the data in your MainActivity
class as shown below:
// In MainActivity class
val prof = Profile(
"Nathan",
23,
true
)
secondActivityIntent.putExtra("profile", prof);
startActivity(secondActivityIntent);
Then retrieve the data in the SecondActivity
class. Remember that you need to cast the object as the data class
type as shown below:
// In SecondActivity class
val p = intent.getSerializableExtra("profile") as Profile
Now you know how to send and receive a Serializable
object between activities, both in Java and Kotlin. Nice work! 👍
Conclusion
The putExtra()
method is used to send data between Android activities.
Any data you put as an extra will be included in the Intent
object received by the next activity you start.
You can retrieve that extra using the get extra methods. For a String
type value, use getStringExtra()
, for a boolean
type value, use getBooleanExtra()
and so on.
To send many values at once, you are recommended to send a Serializable
object instead of repeatedly calling the putExtra()
method.
With this tutorial, you’ve seen how to use putExtra()
both in Kotlin and Java language.
I hope this tutorial has helped you learn how the putExtra()
method works.