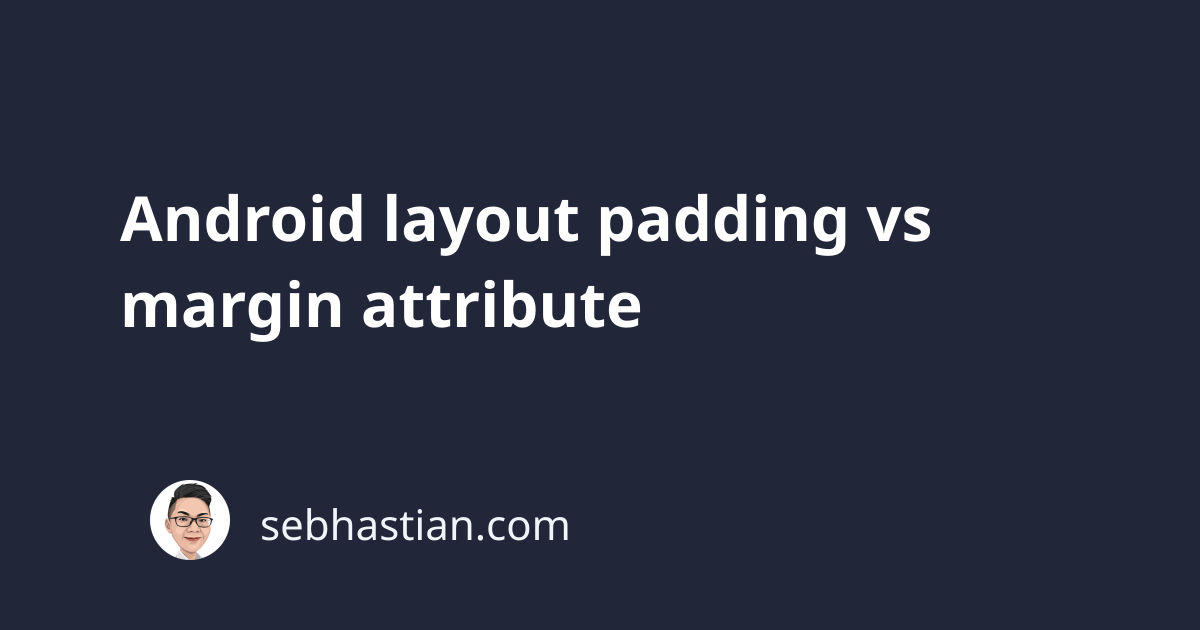
The padding and margin attributes of an Android XML layout are used to add spaces to your view layout.
The padding attributes are used to set the spaces between your content and the border of your widget element.
The margin attributes are used to set the spaces outside of your view.
Here are the padding and margin attributes of a TextView
illustrated:
Let’s see an example of applying paddings and margins in an XML layout.
Suppose you have two TextView
widgets in a LinearLayout
as shown below:
Let’s add the padding
attribute to the TextView1
widget:
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="48dp"
android:text="TextView1" />
You will see the layout change in your design view, with spaces added to the TextView1
widget as follows:
Next, let’s add some margin to the TextView2
widget:
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="24dp"
android:text="TextView2" />
By adding the layout_margin
attribute to the TextView2
widget, you will see the widget appears to have some distance from the TextView1
widget:
This is because a margin of 24dp
has been applied around the TextView2
widget.
And that’s all there is to margins and paddings in Android layout.
Setting the padding
and layout_margin
attribute will add paddings and margins to the four sides of the view where you define the attributes.
You can set individual sides of the view using the attributes provided by Android.
The complete list of padding attributes is as follows:
padding
paddingStart
paddingEnd
paddingTop
paddingRight
paddingBottom
paddingLeft
paddingVertical
paddingHorizontal
These attributes are self-explained by the names they have.
The paddingVertical
is used to set paddings on the top and bottom sides of the view (like applying paddingTop
and paddingBottom
with the same value)
And paddingHorizontal
is used to set the left and right sides of the view.
The paddingStart
and paddingEnd
attributes work like the paddingLeft
and paddingRight
attributes, but they are aware of the letter direction used in your layout.
This means when you use a Right to Left (RTL) locale, the paddingStart
will affect the right side of the view instead of the left.
The same is true for margin attributes:
layout_margin
layout_marginStart
layout_marginEnd
layout_marginTop
layout_marginRight
layout_marginBottom
layout_marginLeft
layout_marginVertical
layout_marginHorizontal
Now you’ve learned the differences between margins and paddings in Android XML layouts. Great work! 👍