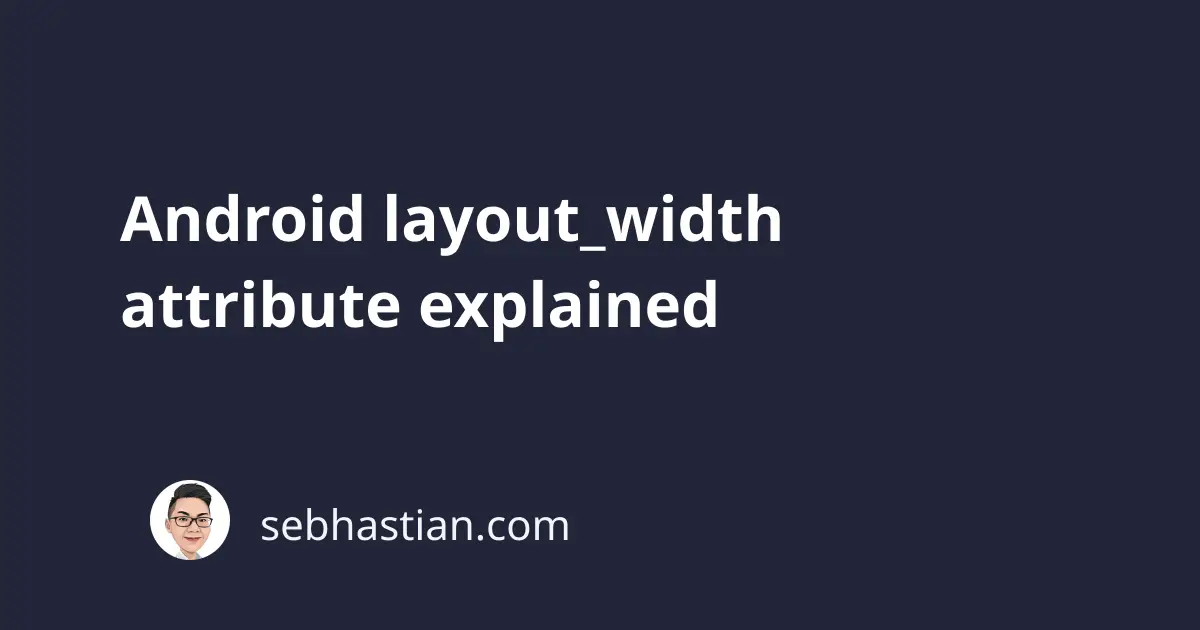
The Android layout_width
attribute is used to define the basic width of a view element you have in your layout.
The width can be specified in any valid dimension measure (dp
, in
, mm
, pt
, sp
, px
)
Or you can also use the special constant for the width: match_parent
and wrap_content
.
Let’s see some examples of the layout_width
attribute in action.
The following code defines the width of a TextView
as 300px
:
<TextView
android:layout_width="300px"
android:background="@color/teal_200"
android:layout_height="wrap_content"
android:text="Hello World!" />
The code above will generate the following output in your layout:
The width of the TextView
element above will be fixed to 300px
.
You can also set the width value to expand or contract depending on the size of the content using the wrap_content
value as follows:
<TextView
android:layout_width="wrap_content"
android:background="@color/teal_200"
android:layout_height="wrap_content"
android:text="Hello World!" />
The wrap_content
value will expand the width of the TextView
element to wrap the content of the element.
Any padding you set in the element will also be taken into account.
The following example shows how to use the wrap_content
value in a TextView
element:
<TextView
android:layout_width="wrap_content"
android:background="@color/teal_200"
android:layout_height="wrap_content"
android:text="Hello World!"/>
The result will be as follows:
You can add horizontal paddings to your view as shown below:
<TextView
android:layout_width="wrap_content"
android:paddingHorizontal="30dp"
android:background="@color/teal_200"
android:layout_height="wrap_content"
android:text="Hello World!"/>
Using the wrap_content
constant, Android will try to set the layout_width
attribute of your views to follow the length of your view’s content.
You can also set the width of your view to follow the width of your view’s parent element using the match_parent
constant.
The match_parent
constant will use the parent’s width excluding the parent’s padding.
Consider the following example:
<TextView
android:layout_width="match_parent"
android:background="@color/teal_200"
android:layout_height="wrap_content"
android:text="Hello World!"/>
The TextView
width should match the parent element as shown below:
If the parent element has paddings, then the width of the view will be reduced by the padding’s value.
In the code below, the LinearLayout
has a horizontal padding of 50dp
:
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingHorizontal="50dp"
tools:context=".MainActivity">
<TextView
android:layout_width="match_parent"
android:background="@color/teal_200"
android:layout_height="wrap_content"
android:text="Hello World!"/>
</LinearLayout>
The code above will produce the following output:
As you can see, paddings in the parent element will reduce the width of the view.
And that’s how the android:layout_width
attribute works. You can try to set various values for the attribute to see how it works for your Android view.