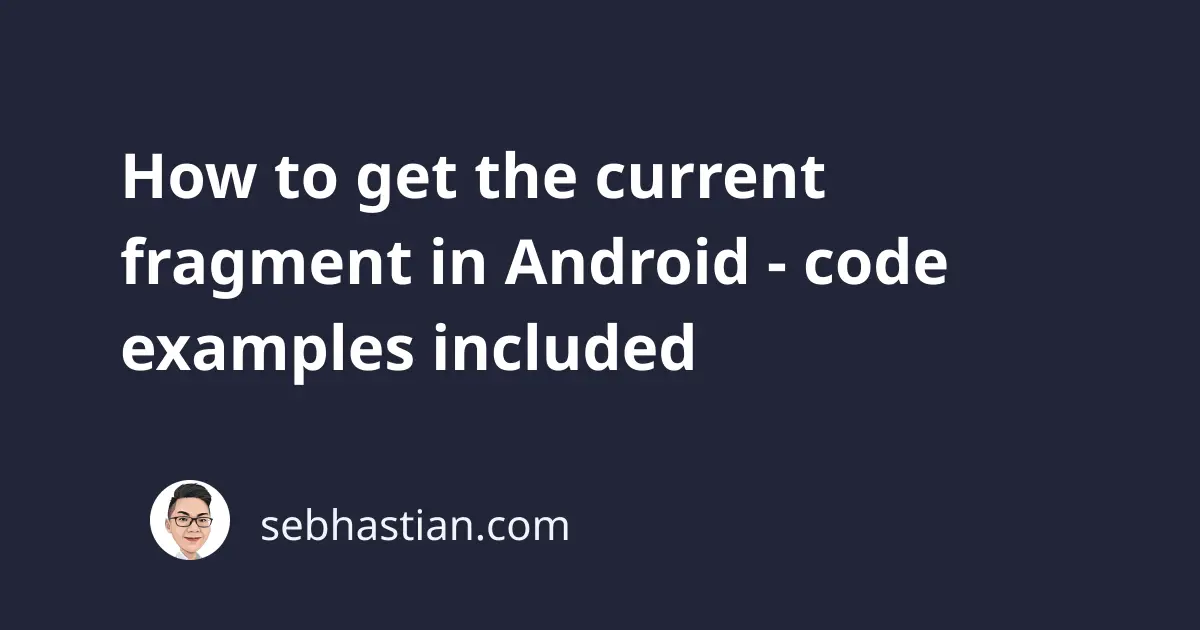
To get the current fragment that’s active in your Android Activity
class, you need to use the supportFragmentManager
object.
The supportFragmentManager
has findFragmentById()
and findFragmentByTag()
methods that you can use to get a fragment instance.
For example, suppose you have a Fragment
class named FirstFragment
created in your activity as shown below:
val fragmentTransaction = supportFragmentManager.beginTransaction()
fragmentTransaction.replace(R.id.frameLayout, FirstFragment())
fragmentTransaction.commit()
In the above example, the FirstFragment
is inflated in a <FrameLayout>
that has an id
value of frameLayout
.
You can get the Fragment
instance inflated inside the frameLayout
using the supportFragmentManager
like this:
supportFragmentManager.findFragmentById(R.id.frameLayout)
In the following example, a click listener was set on the activity button myBtn
.
When the button is clicked, Android will try to find a fragment by the id
value.
Then, check the instance type by using the Kotlin is
operator:
myBtn.setOnClickListener {
val fragmentInstance = supportFragmentManager.findFragmentById(R.id.frameLayout)
if(fragmentInstance is FirstFragment){
Toast.makeText(this, "FirstFragment found", Toast.LENGTH_SHORT).show()
} else {
Toast.makeText(this, "Not found", Toast.LENGTH_SHORT).show()
}
}
When the fragmentInstance
is an instance of the FirstFragment
class, the if
statement will evaluate to true
.
Alternatively, you can also get a fragment by the tag you set in the replace()
method of the fragmentTransaction
instance:
// Set a tag after the fragment class:
fragmentTransaction.replace(R.id.frameLayout, FirstFragment(), "FF1")
The tag FF1
defined above allows you to retrieve the fragment using the FragmentManager.findFragmentByTag()
method:
val fragmentInstance = supportFragmentManager.findFragmentByTag("FF1")
Once you get the current fragment by its tag, you can use the is
operator again to check the fragment’s instance type.
Get the current fragment using Java
When you’re developing an Android application with Java, you can get the current fragment instance using the FragmentManager
class.
You need to find the fragment by the id
of the <FragmentContainerView>
or the <FrameLayout>
tag you used as the container of your fragment.
For example, suppose you placed a Fragment
class named FirstFragment
in your <FragmentContainerView>
as shown below:
<androidx.fragment.app.FragmentContainerView
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/fragment_container_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:name="org.metapx.FirstFragment" />
You can get the FirstFragment
instance programmatically with Java in your Activity
class code.
First, get the FragmentManager
instance by calling the getSupportFragmentManager()
method:
FragmentManager fm = getSupportFragmentManager();
Using the fm
instance, call the findFragmentById()
method and pass the view id
value as its argument.
In this example, the id
value is fragment_container_view
:
Fragment fragInstance = fm.findFragmentById(R.id.fragment_container_view);
The last thing you need to do is check the fragInstance
if it’s an instance of the FirstFragment
as shown below:
if (fragInstance instanceof FirstFragment){
Toast.makeText(
MainActivity.this,
"FirstFragment found",
Toast.LENGTH_SHORT
).show();
}
By using the instanceof
operator, you can check if the fragInstance
you retrieved is an instance of a specific class.
And that’s how you can get the current fragment in an Android application, both in Kotlin and in Java.
Feel free to copy and adjust the code in this tutorial for your Android application. 😉