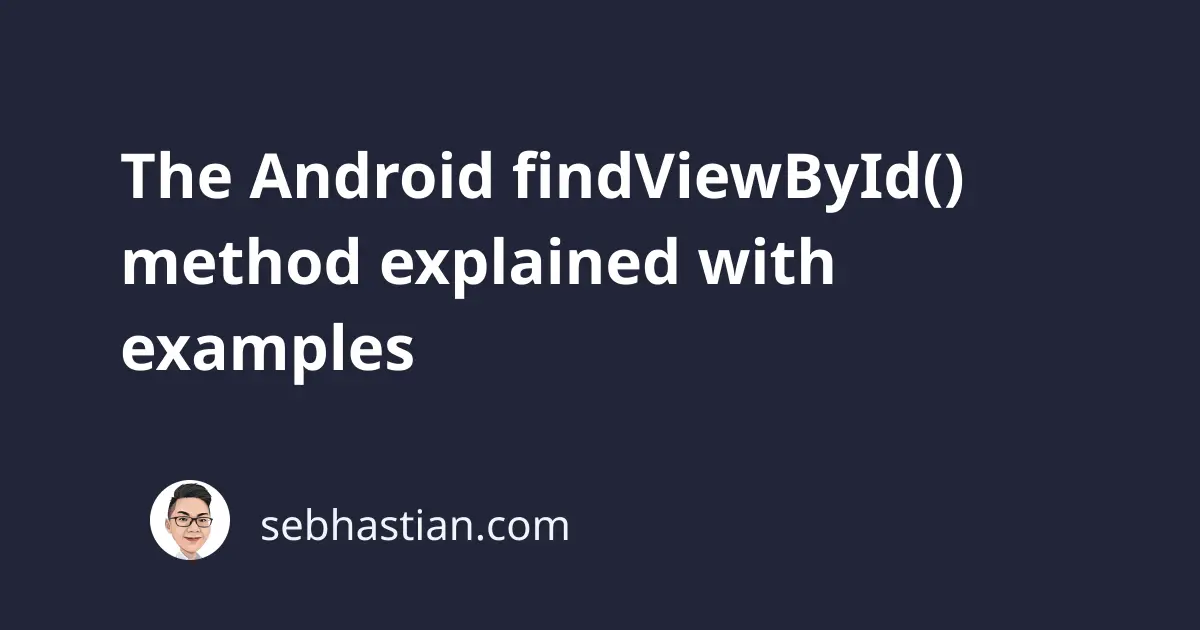
The findViewById()
method is a method of Android’s View
and Activity
classes.
The method is used to find an existing view in your XML layout by its android:id
attribute.
For example, suppose you have a TextView
in your XML layout with the following definition:
<TextView
android:id="@+id/id_text_view"
android:text="Hello World!"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
You can get the TextView
above in your Activity
class with the following code:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TextView myTextView = findViewById(R.id.id_text_view);
}
The same can be done for any valid Android View
object, such as a Button
or a CheckBox
view.
When you call the findViewById()
method, the method is usually called from the AppCompatActivity
class, which is a subclass of the Activity
class.
The findViewById()
method accepts only one parameter, which is the id
of the view with int
type reference:
@Override
public <T extends View> T findViewById(@IdRes int id) {
return getDelegate().findViewById(id);
}
The Android framework generates an internal reference of the id you specified in the XML layout. The internal reference is of an int
type.
You need to refer to this generated int
value by calling R.id
in your code.
Using findViewById in Kotlin
In Kotlin, you can use the syntax to use the findViewById()
method is as follows:
val myTextView = findViewById<TextView>(R.id.id_text_view)
You need to specify the generic type of the view inside the angle brackets <>
as shown above.
And that’s how you use the findViewById()
method when developing an Android project. 👍