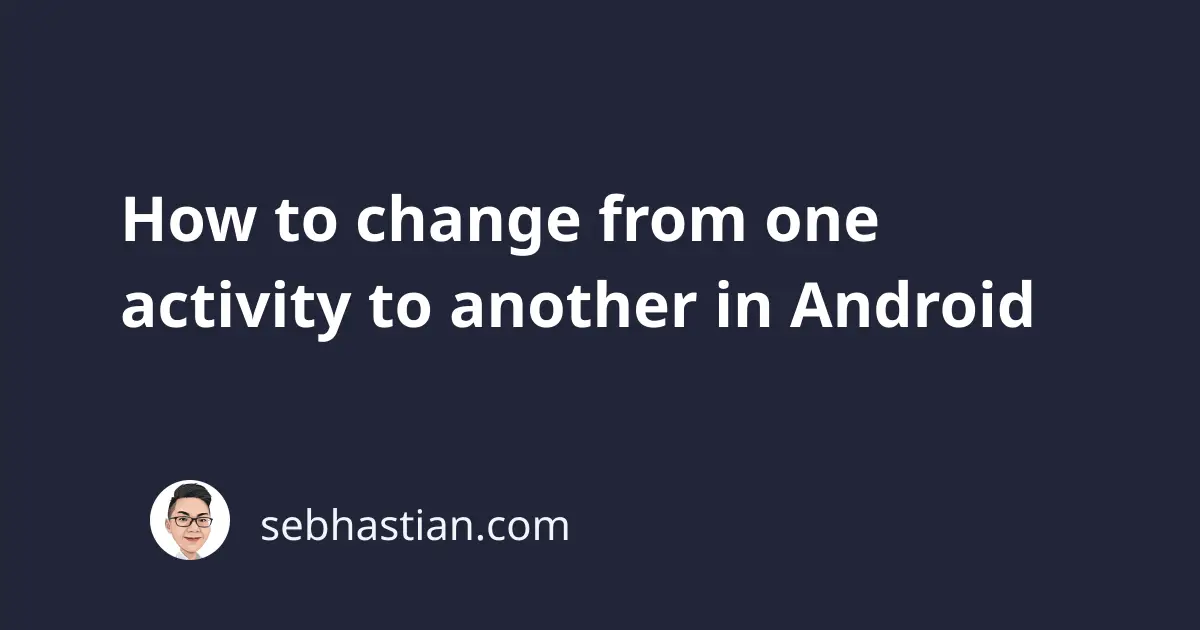
The Android Activity
class is used to draw the user interface on the screen.
Usually, a complete Android application consists of multiple activities. One Activity
serves as the main entry point when you open the app from your home screen.
Another Activity
will be executed when you need to do a specific task, and so on.
With multiple activities defined in your application, you need a way to move between the activities.
To change the currently active Activity
in your application, you need to run the startActivity()
method from the Activity
class.
For example, here’s the code to move from MainActivity
to SecondActivity
in Java:
Intent secondActivityIntent = new Intent(this, SecondActivity.class);
startActivity(secondActivityIntent);
The two lines code above is all you need to move from one activity to another.
You can try the code above by placing it in the onCreate()
method of the MainActivity
class:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Intent secondActivityIntent = new Intent(
this, SecondActivity.class
);
startActivity(secondActivityIntent);
}
}
Make sure that you have an activity named SecondActivity
in your project first. An empty activity will do.
When you launch the application, the screen will automatically move to the SecondActivity
.
To see the change, let’s create the Intent
object and run the startActivity()
method when a Button
is clicked.
Here’s the code for the MainActivity
class:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button buttonSecondActivity = findViewById(
R.id.button_second_activity
);
buttonSecondActivity.setOnClickListener(view -> {
Intent secondActivityIntent = new Intent(
getApplicationContext(), SecondActivity.class
);
startActivity(secondActivityIntent);
});
}
}
And here’s the code for the activity_main.xml
file:
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button_second_activity"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Move to Second Activity"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Next, let’s create the SecondActivity
class with the following code:
public class SecondActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
Button buttonMainActivity = findViewById(
R.id.button_main_activity
);
buttonMainActivity.setOnClickListener(view -> {
Intent mainActivityIntent = new Intent(
getApplicationContext(), MainActivity.class
);
startActivity(mainActivityIntent);
});
}
}
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".SecondActivity">
<Button
android:id="@+id/button_first_activity"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Move to Main Activity"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Now that the code is complete, run the application.
You will see that the activity will change when you tap on the button. You can also return to the previous activity by tapping the Back button on your device.
You can see the full code in Java language here:
nsebhastian/JavaChangeActivity
For Kotlin, the repository can be found here:
nsebhastian/KotlinChangeActivity
Now you’ve learned how to change from one activity to another in Android. Good work! 👍