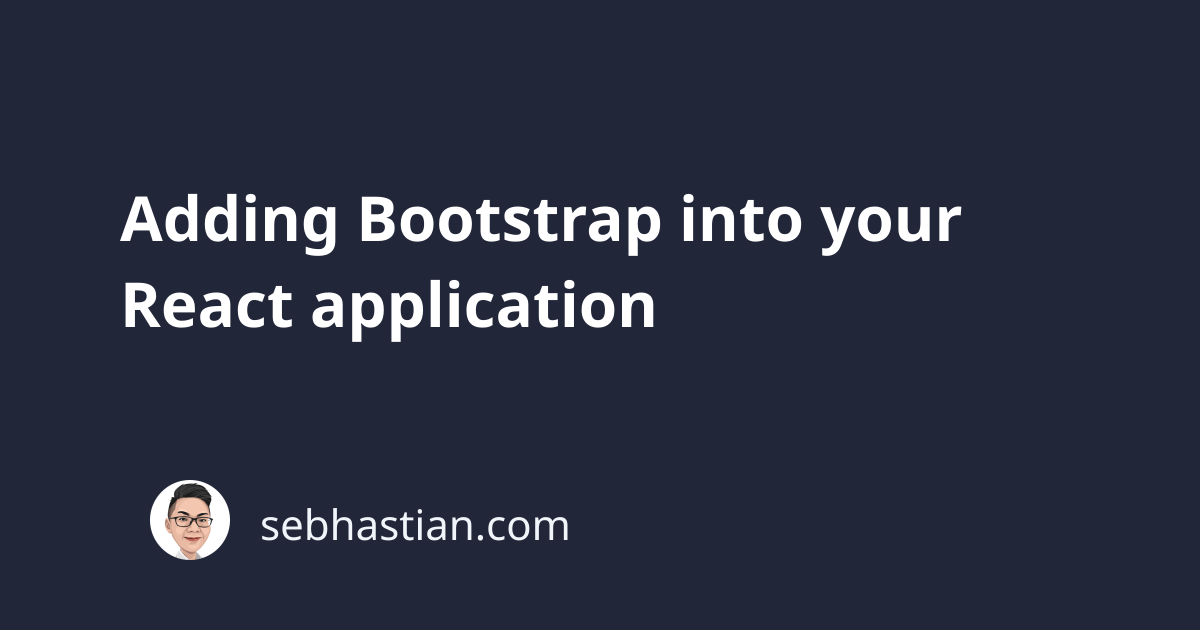
Can Bootstrap be used with React?
How do I import Bootstrap into React?
There are 3 common ways you can use Bootstrap in your React project:
- Using Bootstrap CDN links
- Using Bootstrap npm package
- Using Bootstrap-React component library
Using Bootstrap CDN links
You can include the compiled CSS and JS files by using BootstrapCDN links into your public/index.html
file:
<!-- CSS only -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous">
<!-- JS, Popper.js, and jQuery -->
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/umd/popper.min.js" integrity="sha384-9/reFTGAW83EW2RDu2S0VKaIzap3H66lZH81PoYlFhbGU+6BZp6G7niu735Sk7lN" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script>
Include Bootstrap CSS link inside your <head>
element:
<head>
<!-- other head attributes -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous">
</head>
Then put the JS links inside your HTML body, just before the closing </body>
tag:
<!-- JS, Popper.js, and jQuery -->
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/umd/popper.min.js" integrity="sha384-9/reFTGAW83EW2RDu2S0VKaIzap3H66lZH81PoYlFhbGU+6BZp6G7niu735Sk7lN" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script>
</body>
And you can use Bootstrap’s classes in your component’s className
:
<button className="btn btn-primary btn-lg">Styled with Bootstrap</button>
Using Bootstrap npm package
Bootstrap has library package that you can install using npm or Yarn:
npm install bootstrap
# or
yarn add bootstrap
Then import Bootstrap CSS file in your root src/index.js
file. Make sure you import the css on the first line to make it applied into all other imported components:
import 'bootstrap/dist/css/bootstrap.css';
You can then style your React components by including the right class names:
<button className="btn btn-primary btn-lg">Click me!</button>
You also need to install jQuery if you want to use Bootstrap’s JavaScript plugins like modal, tooltip, or alert.
Import Bootstrap’s JavaScript bundle below the CSS import:
import 'bootstrap/dist/css/bootstrap.min.css';
import 'bootstrap/dist/js/bootstrap.bundle.min';
Next, install jQuery using npm or Yarn:
npm install jquery
# or
yarn add jquery
Now you can trigger a modal using a button:
import React from "react";
function App() {
return (
<>
<button
type="button"
className="btn btn-primary"
data-toggle="modal"
data-target="#exampleModal"
>
Launch demo modal
</button>
<div
className="modal fade"
id="exampleModal"
tabIndex="-1"
role="dialog"
aria-labelledby="exampleModalLabel"
aria-hidden="true"
>
<div className="modal-dialog" role="document">
<div className="modal-content">
<div className="modal-header">
<h5 className="modal-title" id="exampleModalLabel">
Modal title
</h5>
<button
type="button"
className="close"
data-dismiss="modal"
aria-label="Close"
>
<span aria-hidden="true">×</span>
</button>
</div>
<div className="modal-body">Hey, you just triggered this modal with a button!</div>
<div className="modal-footer">
<button
type="button"
className="btn btn-secondary"
data-dismiss="modal"
>
Close
</button>
<button type="button" className="btn btn-primary">
Save changes
</button>
</div>
</div>
</div>
</div>
</>
);
}
export default App;
Using the Bootstrap-React component library
Aside from importing Bootstrap and styling your components with className(s) manually, you can use a Bootstrap-React component library that wraps the Bootstrap jQuery plugin inside React components. React Bootstrap and Reactstrap are component libraries that allows you to customize its style and behavior using props and state.
The differences between both libraries are subjective to the user’s experience, because both serve the same purpose. They also require you to install Bootstrap as a dependency:
npm install bootstrap react-bootstrap
# or
npm install bootstrap reactstrap
You can then import any of the available components into your React project and implement the style using props. No need to import any CSS or JS file into the src/index.js
file.
Here’s how you do it in React Bootstrap:
import Button from 'react-bootstrap/Button';
<Button variant="primary">Primary</Button>
and in Reactstrap:
import { Button } from 'reactstrap';
<Button color="primary">primary</Button>
You can get further information on both React Bootstrap and Reactstrap’s documentation sites.