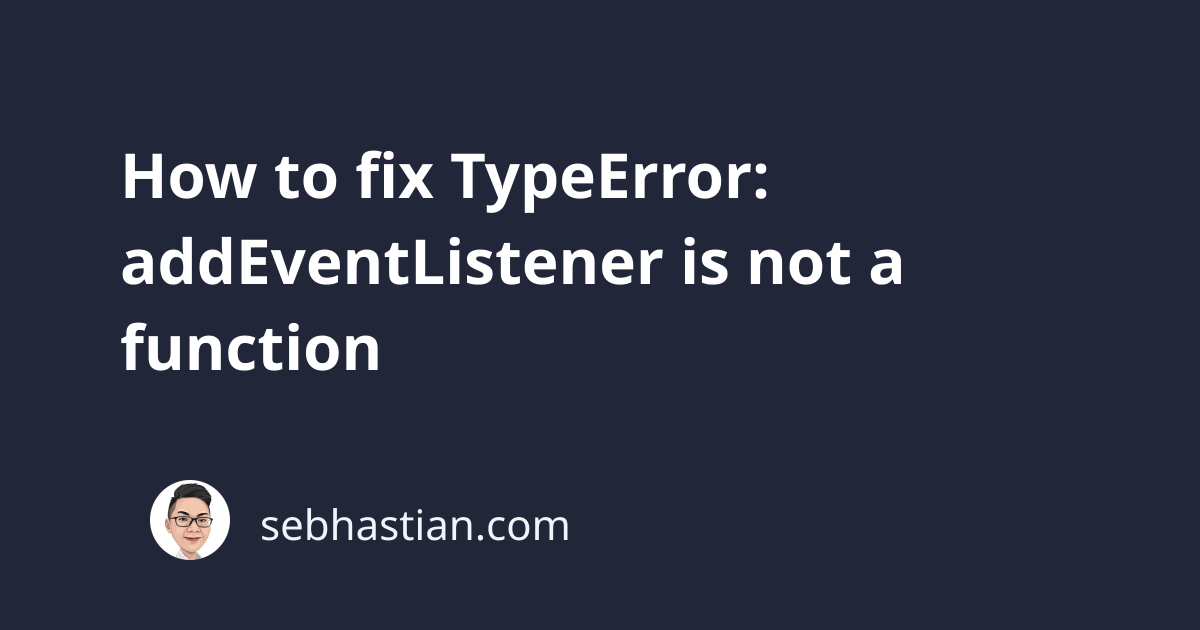
When running JavaScript code, you might encounter the error below:
TypeError: addEventListener is not a function
This error occurs when you call the addEventListener()
method from an object that doesn’t know about the method.
Most likely, you’re calling the method not from an Element
object but from an array instead.
Let’s see an example that causes this error and how to fix it in practice.
How to reproduce the error
Suppose you have an HTML document with the following content:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Nathan Sebhastian</title>
</head>
<body>
<div class="reg">Line 1</div>
<div class="reg">Line 2</div>
<div class="reg">Line 3</div>
</body>
</html>
Next, you run the following JavaScript code in a <script>
tag:
const divEl = document.getElementsByClassName("reg");
divEl.addEventListener("click", function onClick() {
alert("line clicked");
});
But when running the code above, you get the following error:
Uncaught TypeError: divEl.addEventListener is not a function
The error happens because the getElementsByClassName()
method returns an HTMLCollection
array object no matter if there’s any matching Element
object or not.
You can’t call the addEventListener()
method from the returned array. You need to iterate over the array using the for .. of
loop like this:
const divEl = document.getElementsByClassName("reg");
for (const element of divEl) {
element.addEventListener("click", function onClick() {
console.log("line clicked");
});
}
Here, the for .. of
loop will iterate over the returned divEl
array and attach an event listener to each Element
object.
If you want to add an event listener only to a specific element in the array, you can access the individual object using the index notation.
The example below shows how to add the event listener only to the ‘Line 2’ element:
const divEl = document.getElementsByClassName("reg");
divEl[1].addEventListener("click", function onClick() {
console.log("line clicked");
});
Here, we accessed a specific element with divEl[1]
to attach the event listener. Because it’s a valid Element
object, the code runs without any error.
The following JavaScript selector methods return an array, so the addEventListener is not a function
error would likely occur when you use any one of these:
getElementsByClassName()
getElementsByName()
getElementsByTagName()
querySelectorAll()
While these selector methods return a valid Element
object or null
when not found:
getElementById()
querySelector()
Knowing the different return values between the selector methods should help you prevent the error from happening in the first place.
Also note that JavaScript methods are case-sensitive, which means you need to type the method exactly as addEventListener
and not addeventlistener
.
I hope this tutorial helps you fix the error. Happy coding! 👍