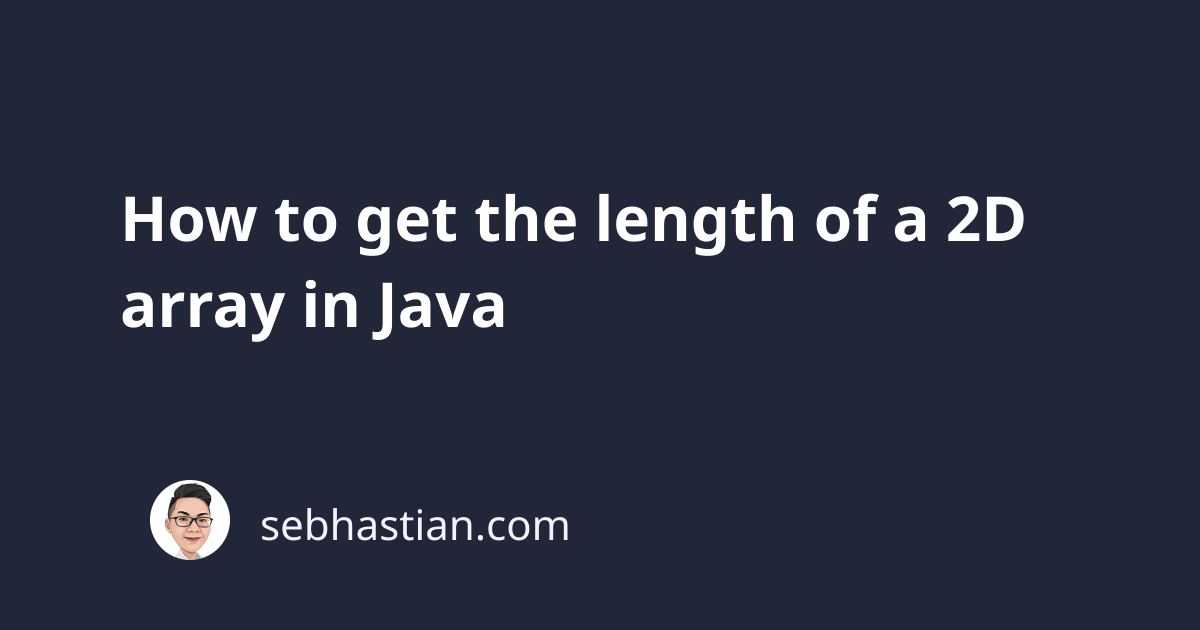
To get the length of a 2D array in Java, you can use the length
field of the array.
The length
field is a property of the array object that returns the number of rows in the array.
Here is an example of how to get the length of a 2D array in Java:
int[][] array = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int numRows = array.length;
int numColumns = array[0].length;
System.out.println("The array has " + numRows + " rows "
+ "and " + numColumns + " columns.");
The code above gets the length
of the array and the first row of that array.
The output is as follows:
The array has 3 rows and 3 columns.
But since a 2D array can have different number of columns for each row, you probably want to find out how many columns each row has.
To get the length
of each array, you need to use a for
loop as shown below:
// create an array of different lengths
int[][] array = {{1, 2, 3}, {4}, {7, 8}};
int numRows = array.length;
System.out.println("The array has " + numRows + " rows.");
// loop over the array
for(int i=0; i<array.length; i++) {
int rowNumber = i+1;
System.out.println("row " + rowNumber + " has " + array[i].length + " columns");
}
The output:
The array has 3 rows.
row 1 has 3 columns
row 2 has 1 columns
row 3 has 2 columns
And that’s how you find the length of a 2D array in Java.